EMOJI
#
Module emoji
is for request data of emoji from
unicode.org site and
store all emoji data inside .josn file on this Python package as
dictionary.
Important
This module only get data from Full Emoji List and Full Emoji Modifiers links.
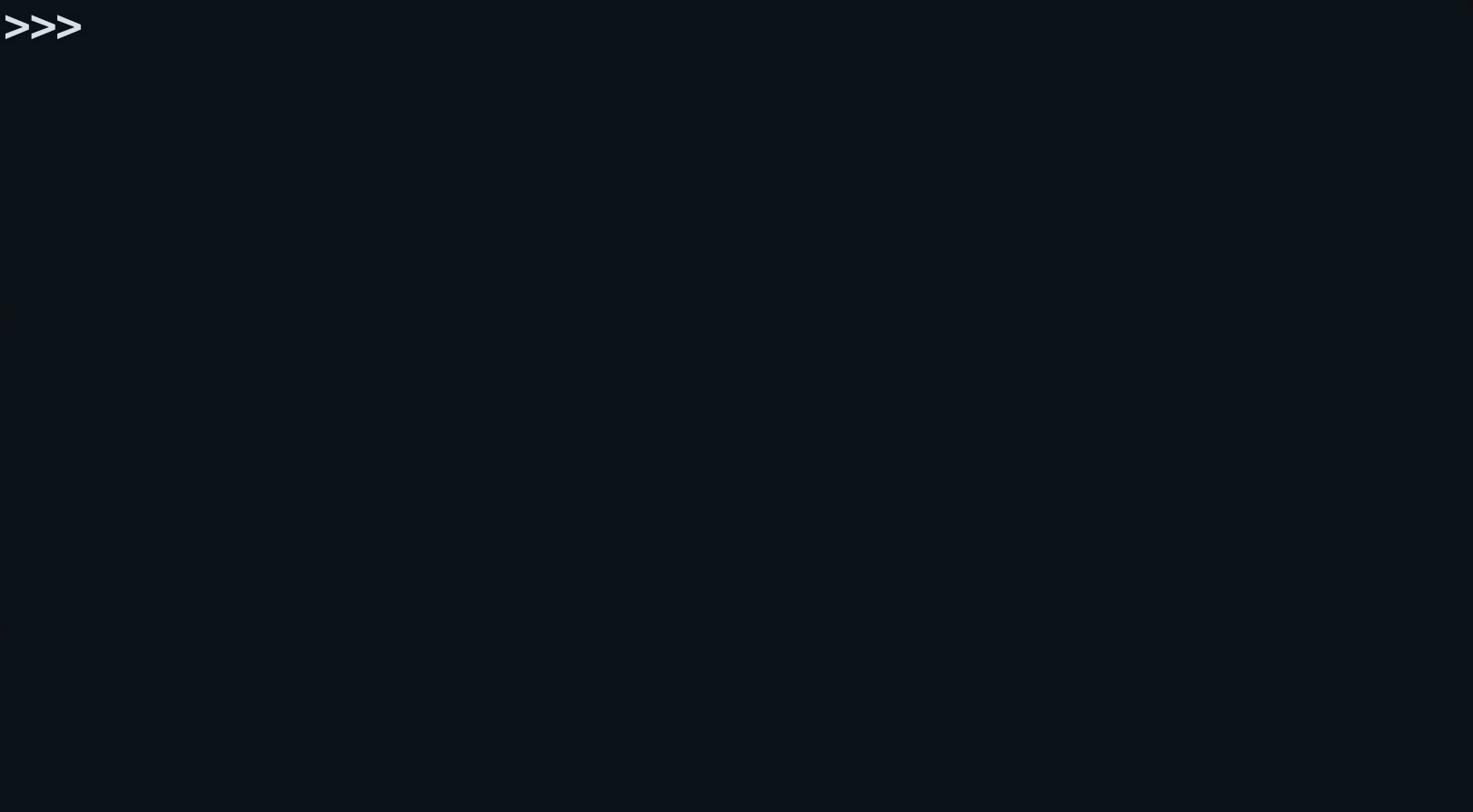
FUNCTION emoji_get_url_data
#
- mdsanima_dev.emoji.emoji_get_url_data(emoji_url: str) str #
This function request emoji data url and waiting raise for status. If status ok returning data text for further processing.
Warning
This function only used for request data in emoji_clean_url_data function.
- Parameters:
emoji_url (str) – Link to emoticons.
- Returns:
HTML code as string text.
- Return type:
str
- Usage:
Assigning a function by calling to a variable:
emoji_url_data = emoji_get_url_data(emoji_lis_url)
FUNCTION emoji_clean_url_data
#
- mdsanima_dev.emoji.emoji_clean_url_data(bhead: bool = False, mhead: bool = False, ucode: bool = False, emoji: bool = False, ename: bool = False, emoji_url: str = 'http://www.unicode.org/emoji/charts/full-emoji-list.html') dict #
This funciton is used to clean up previously requested data and store in dictionary
.json
file and saved in tojson
folder. These folder also included in Python package. Default save a first linkemoji_lis_url
, the second link is theemoji_mod_url
which are included in this module as a variable. If you need to download new data, you can use just these two variables only.You can use parameter to debug what data is saved. By defaults for the end this function printing only stats in colors. If you want to see use debug parameters. Debug mode show cool colors from extracting url.
Warning
This two link is important, please check this in the beginning on this page.
- Parameters:
bhead (bool, optional) – Debug mode show big head, defaults to
False
.mhead (bool, optional) – Debug mode show medium head, defaults to
False
.ucode (bool, optional) – Debug mode show unicode, defaults to
False
.emoji (bool, optional) – Debug mode show emoji, defaults to
False
.ename (bool, optional) – Debug mode show emoji name, defaults to
False
.emoji_url (str, optional) – Link to extract data, defaults to
emoji_lis_url
.
- Returns:
Save dictionary data in to
.json
file.- Return type:
dict
- Usage:
Function call extract and save defaults data in debug mode and second example also save second ling in debug mode:
emoji_clean_url_data(bhead=True) emoji_clean_url_data(emoji_url=emoji_mod_url, mhead=True)
FUNCTION emoji_load_json_data
#
- mdsanima_dev.emoji.emoji_load_json_data() dict #
This function loading the json data generated on previous emoji_clean_url_data function.
- Returns:
Dictionary json on all emoji with joining two json
emoji_list
andemoji_modifiers
.- Return type:
dict
- Usage:
Assigning a function by calling to a variable:
emo = emoji_load_json_data()
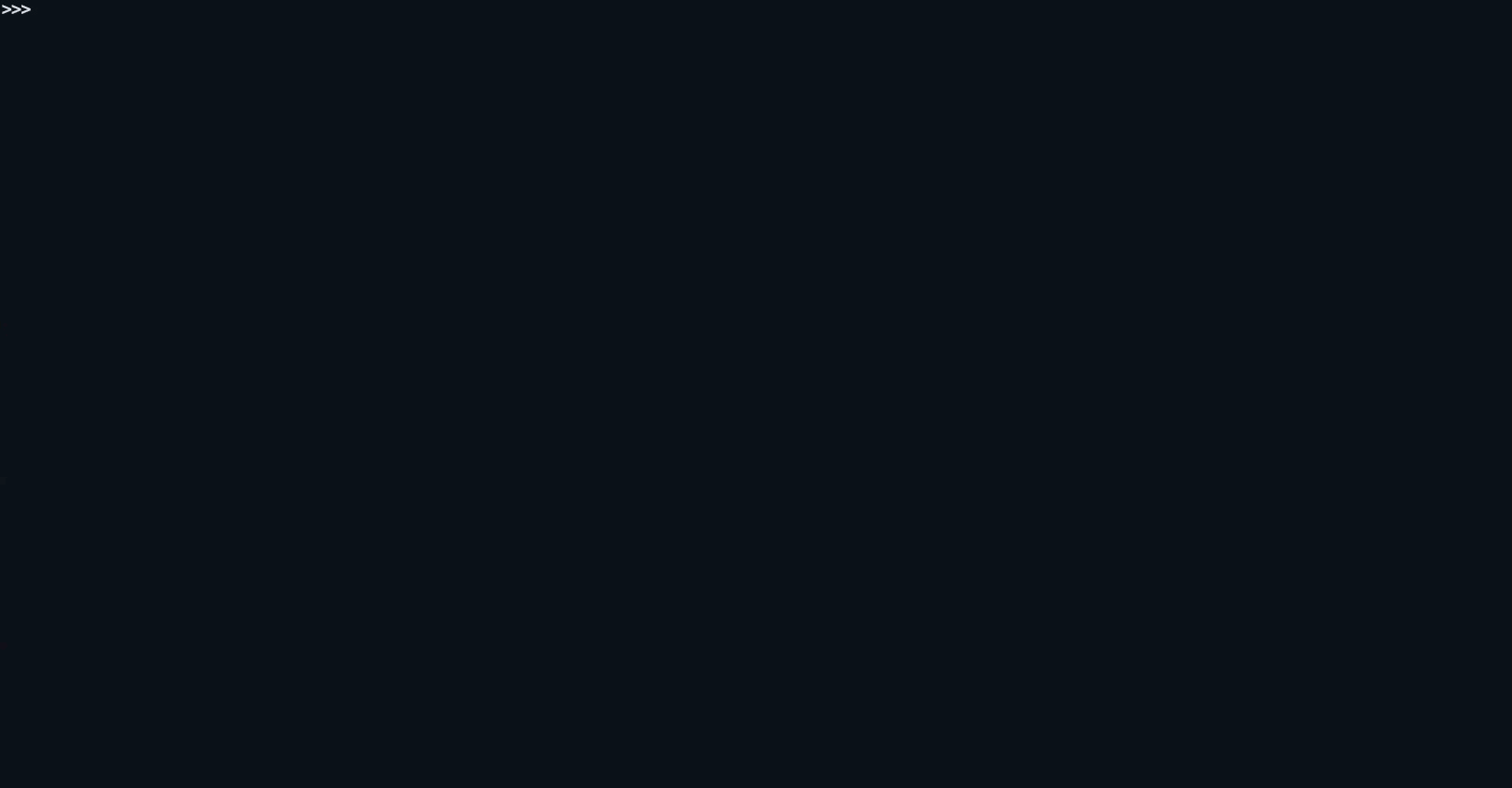
CLASS emoji
#
- class mdsanima_dev.emoji.emoji#
This class has methods for printing various emoji functions.
Warning
Class show print all emoji with calling methods inside this class. We’re recommended used only class show(emoji) to print big head, medium head, or just only one emoji.
- emo_all(number: bool = False, names: bool = False) str #
This method prints all available emojis, sorted by heads. You can use arguments to print number of emoji and names of emoji.
- Parameters:
number (bool, optional) – Print emoji numbers, defaults to
False
.names (bool, optional) – Print emoji names, defaults to
False
.
- Returns:
Prints all available emojis.
- Return type:
str
- emo_big_head() str #
This method printing all big head text value of emoji in the table with colored table and colored text.
- emo_head(bhead: str, mhead: str, number: bool = False, names: bool = False) str #
This method printing all emoji in to a given group.
- Parameters:
bhead (str) – Name of big head emoji.
mhead (str) – Name of medium head emoji.
number (bool, optional) – Print emoji numbers, defaults to
False
.names (bool, optional) – Print emoji names, defaults to
False
.
- Returns:
Printing all emoji in to a given group.
- Return type:
str
- emo_medium_head() str #
This method printing all medium head text value of emoji in the table with colored table and colored text.
- emo_stats() str #
This method printing the statistic of emoji in the table with colored table and colored text.
- emoji(number: int, end: str = '') str #
Printing only one emoji.
- Parameters:
number (int) – Emoji number to printing.
end (str, optional) – Ends of print, defaults to empty string, that’s set a print two or more emoji in the same line.
- Returns:
Print one emoji.
- Return type:
str
CLASS show
#
- class mdsanima_dev.emoji.show(emo_list: str)#
This class show all emoji or just only one. See documentation for more information and screenshot.
- Parameters:
emoji (class) – Master class of emoji.
- Usage:
Assigning a function by calling to a variable:
show_me = show("emoji_list") show_me.emo_stats() show_me.emo_big_head() show_me.emo_medium_head() show_me.emo_all(True, False) show_me.emo_head("objects", "music", True, True) show_me.emoji(986)
Calling method with arguments in to class:
show("emoji_modifiers").emo_head("people_body", "hands")
Now I will show you how to use class show(emoji) method to find out what number each emoji has so that you can use in your code:
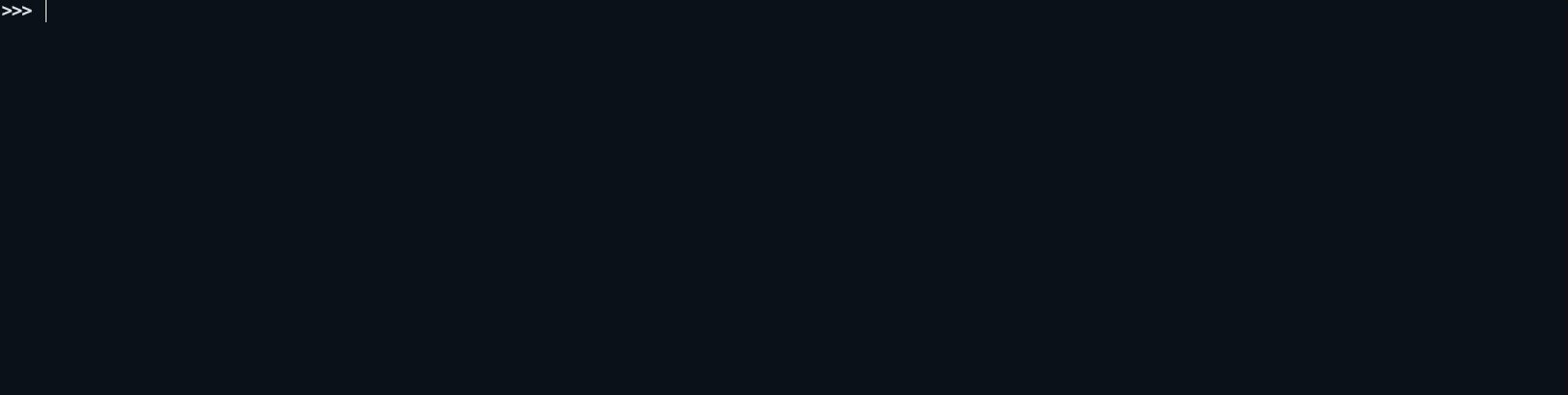
METHOD emo_stats()
#
This method show statistic about emoji published on .json files which was retrieved on the emoji_clean_url_data function. You can use emoji_list or emoji_modifiers only:
from mdsanima_dev.emoji import show
s = show("emoji_list")
s.emo_stats()
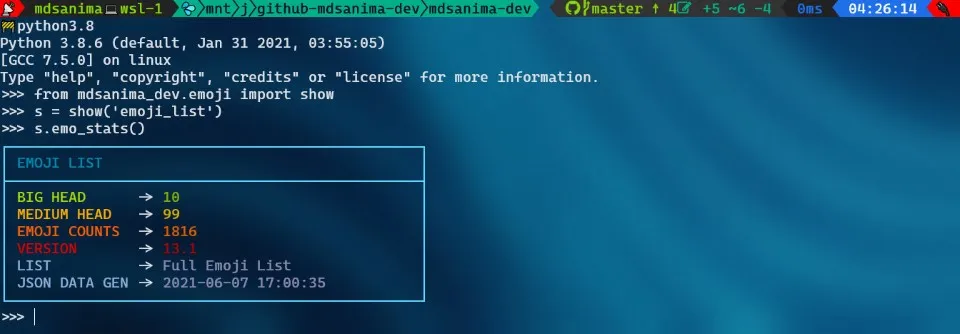
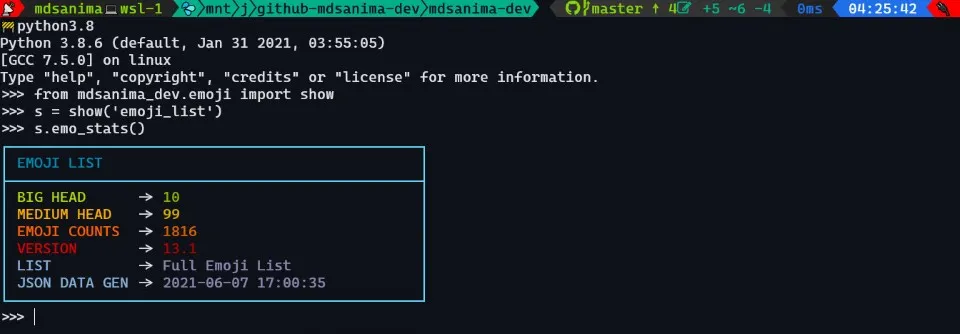
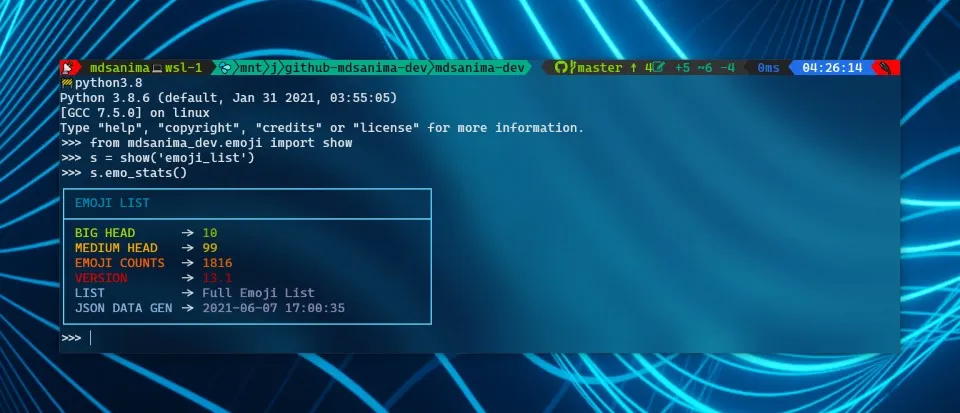
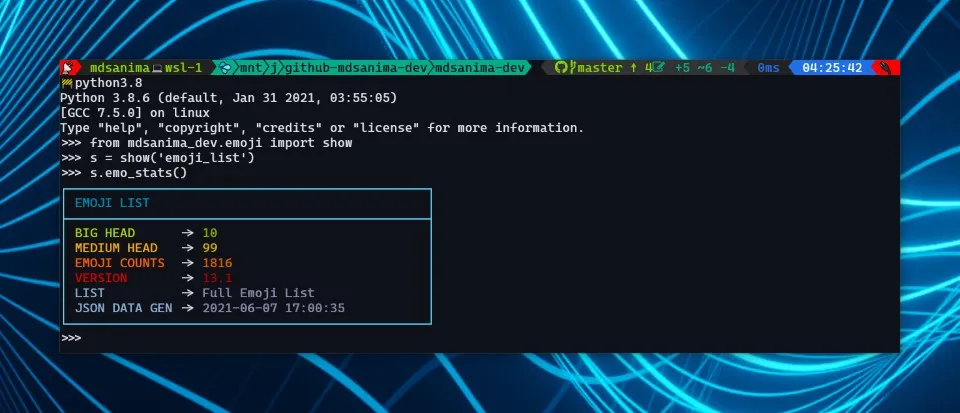
METHOD emo_all()
#
This method will display all the available emoji from the list you have selected. All emoji in the given group will be displayed on one line:
from mdsanima_dev.emoji import show
s = show("emoji_list")
s.emo_all()
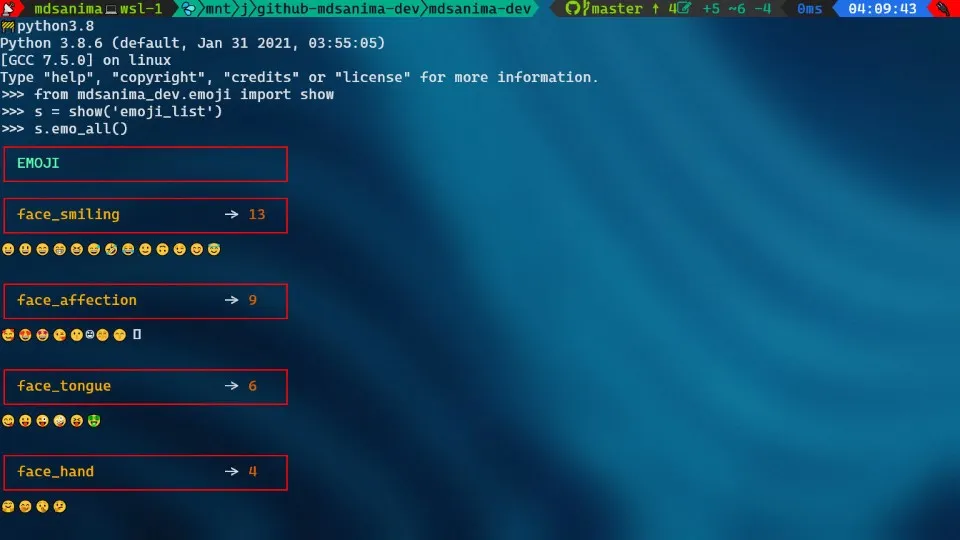
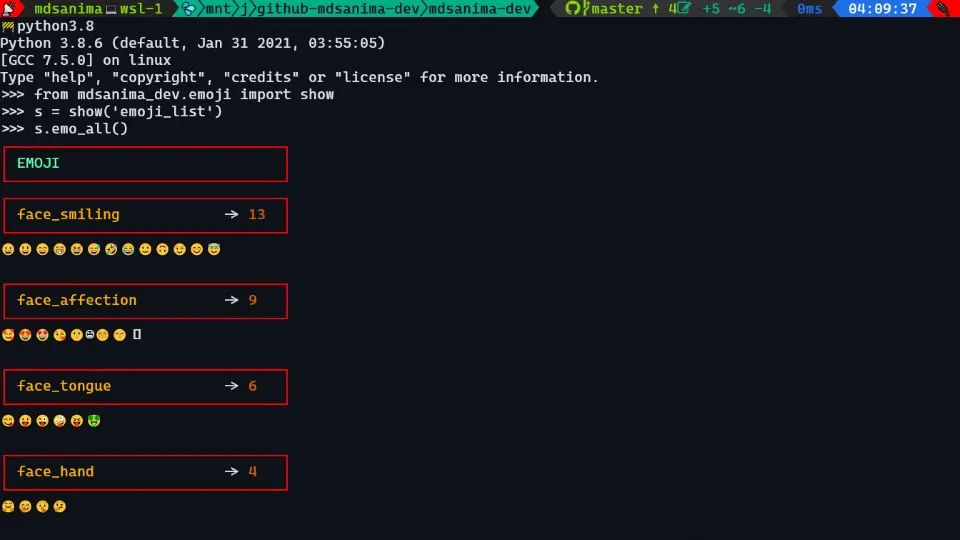
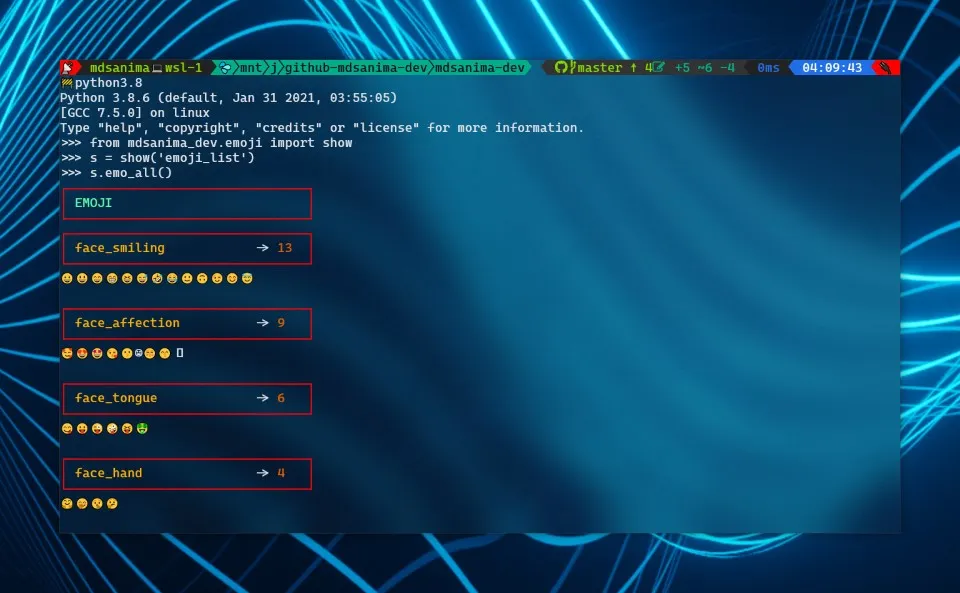
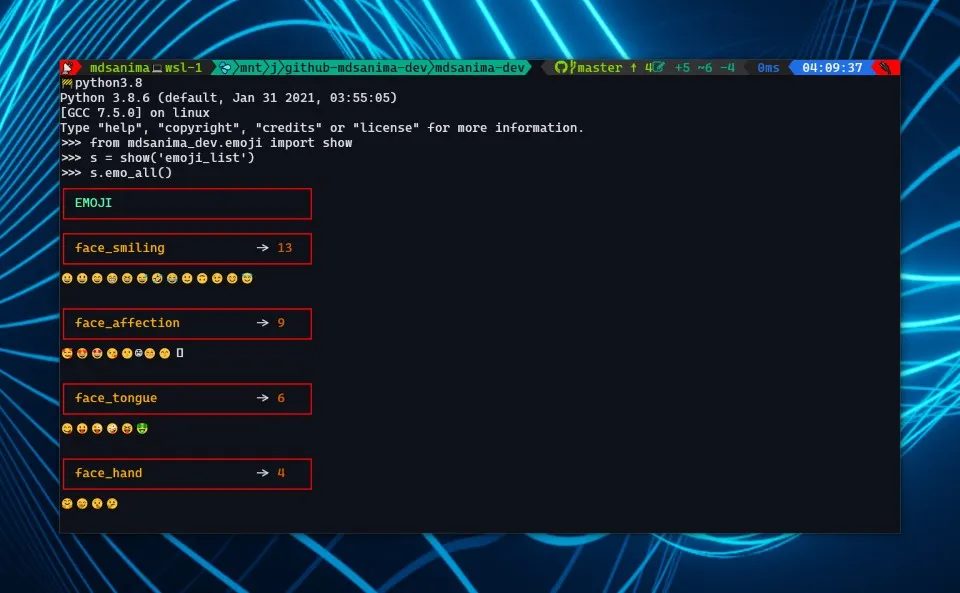
METHOD emo_all(True, False)
#
The first argument is true so it will show us all the emoji and a number that is assigned to the emoji. All emoji in the given group will be displayed on one line:
from mdsanima_dev.emoji import show
s = show("emoji_list")
s.emo_all(True, False)
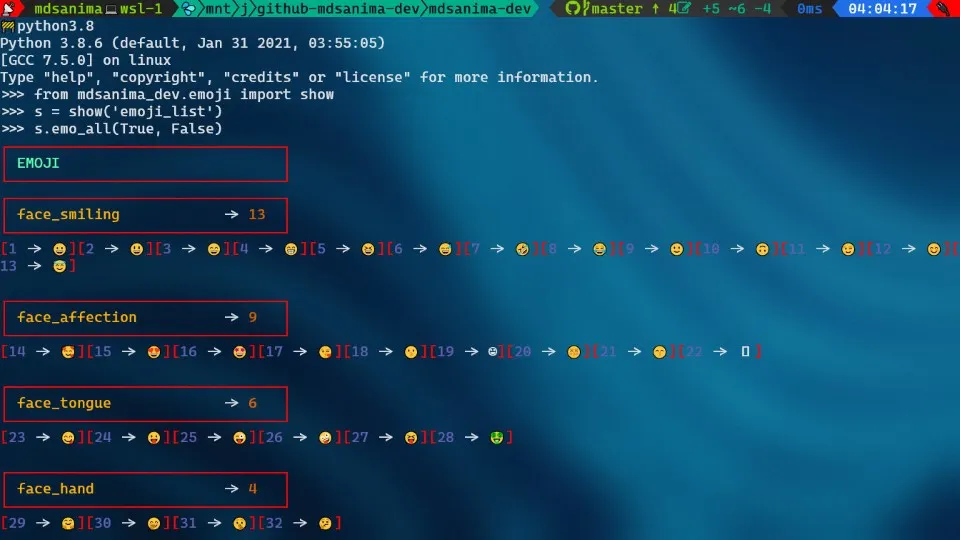
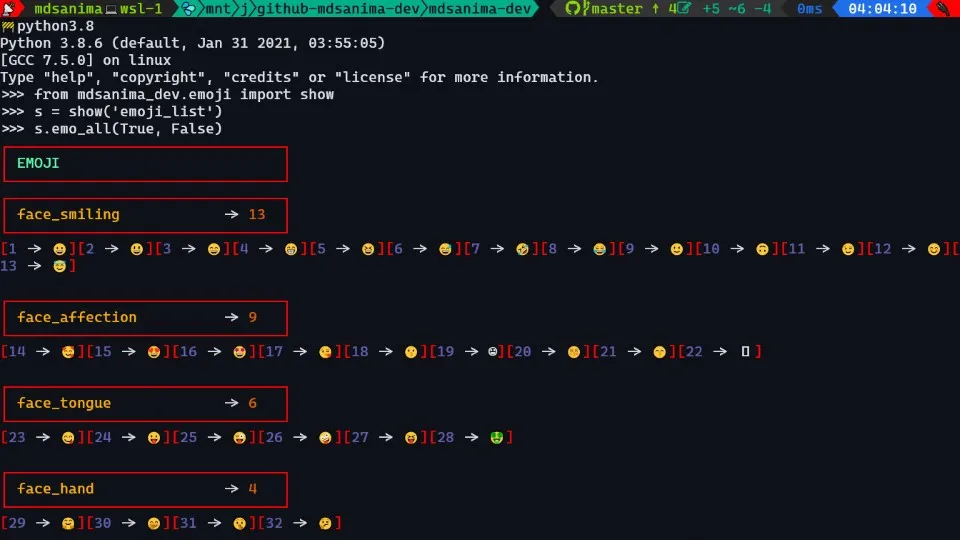
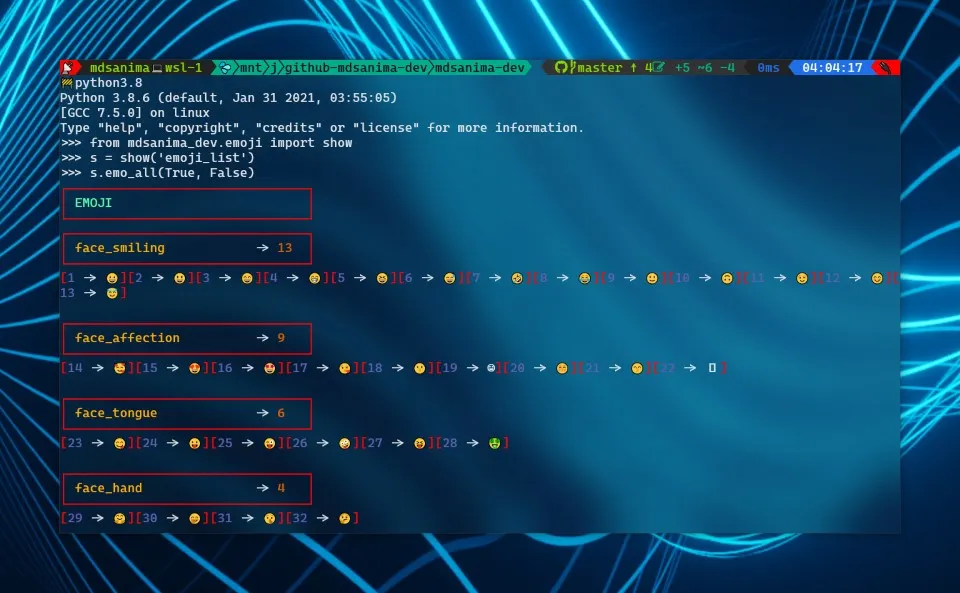
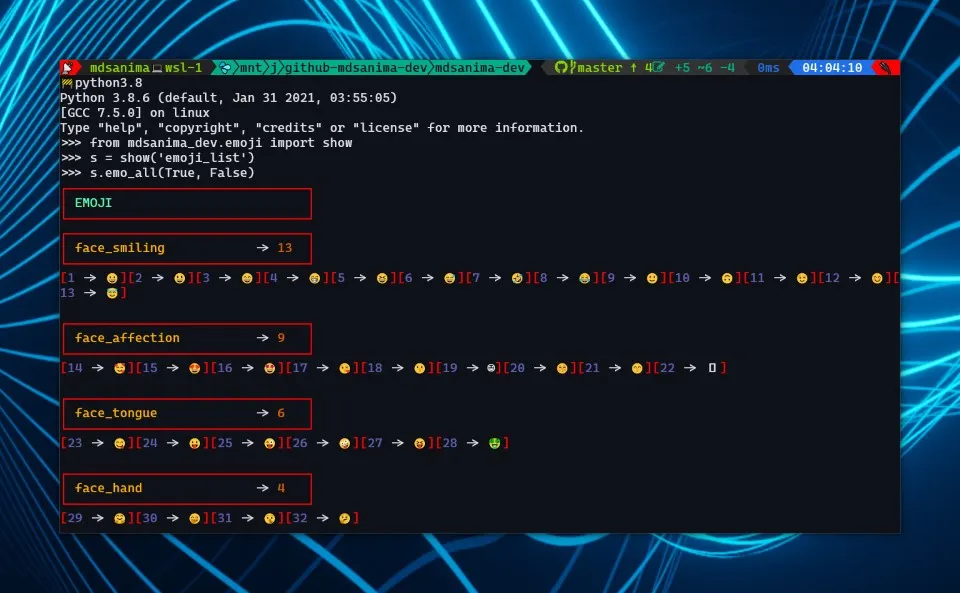
METHOD emo_all(False, True)
#
The second argument is true so it will show us all the emojis and a name thats is assigned to the emoji. All emoticons in a given group will be displayed individually, each on a separate line:
from mdsanima_dev.emoji import show
s = show("emoji_list")
s.emo_all(False, True)
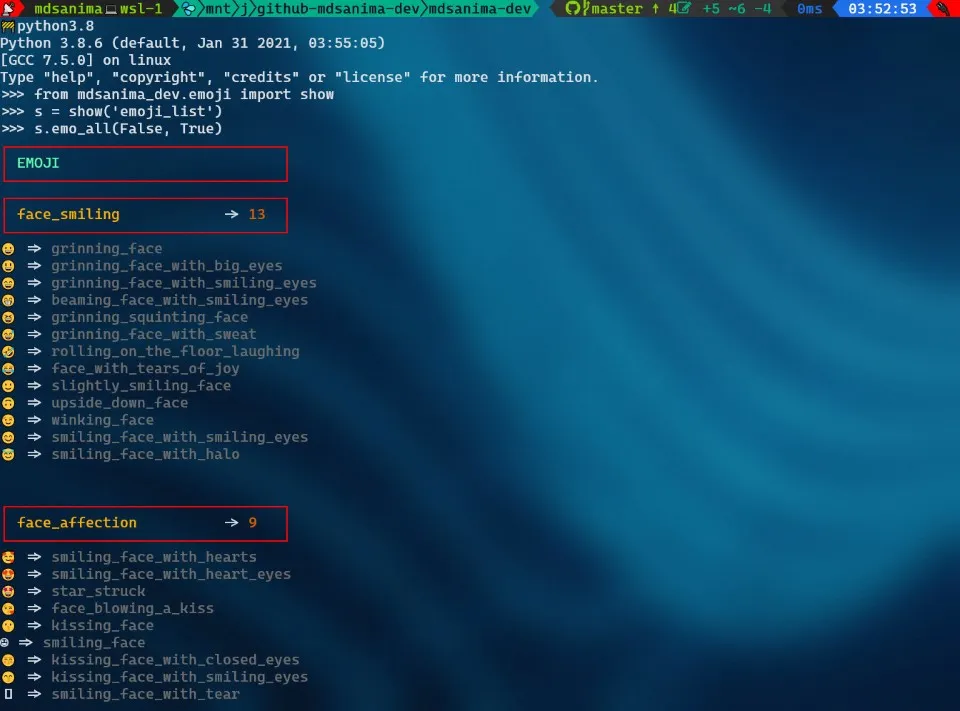
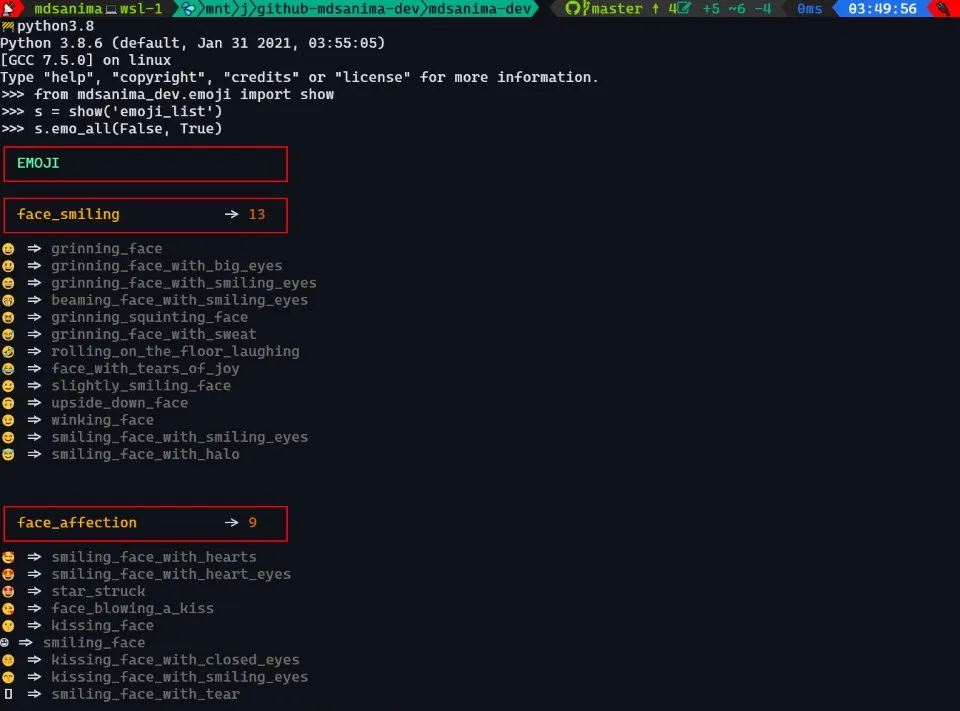
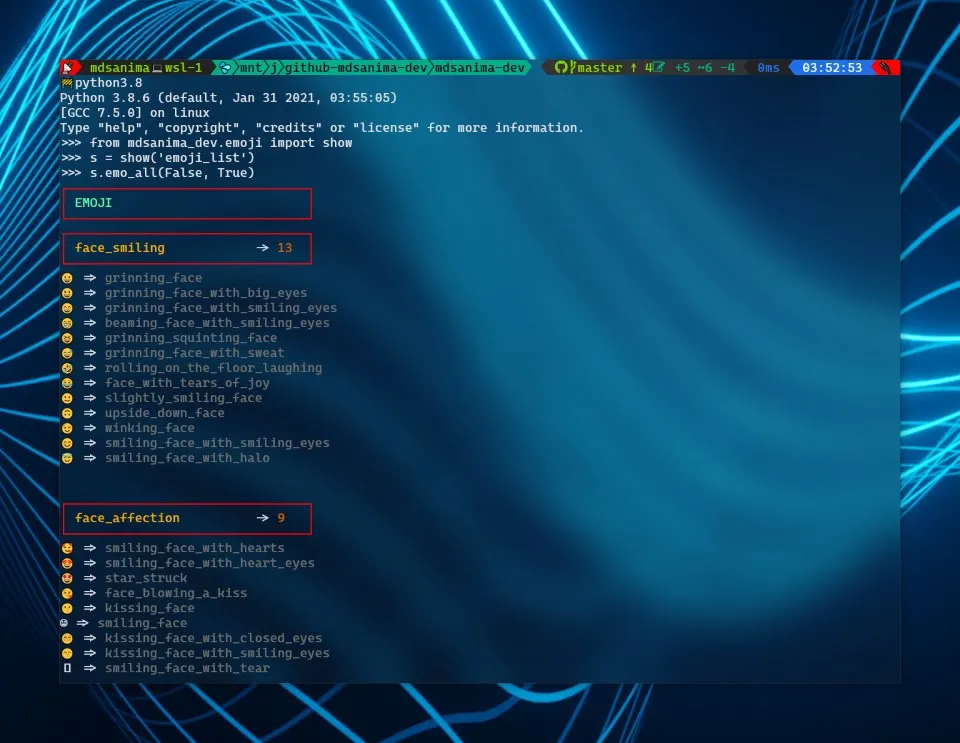
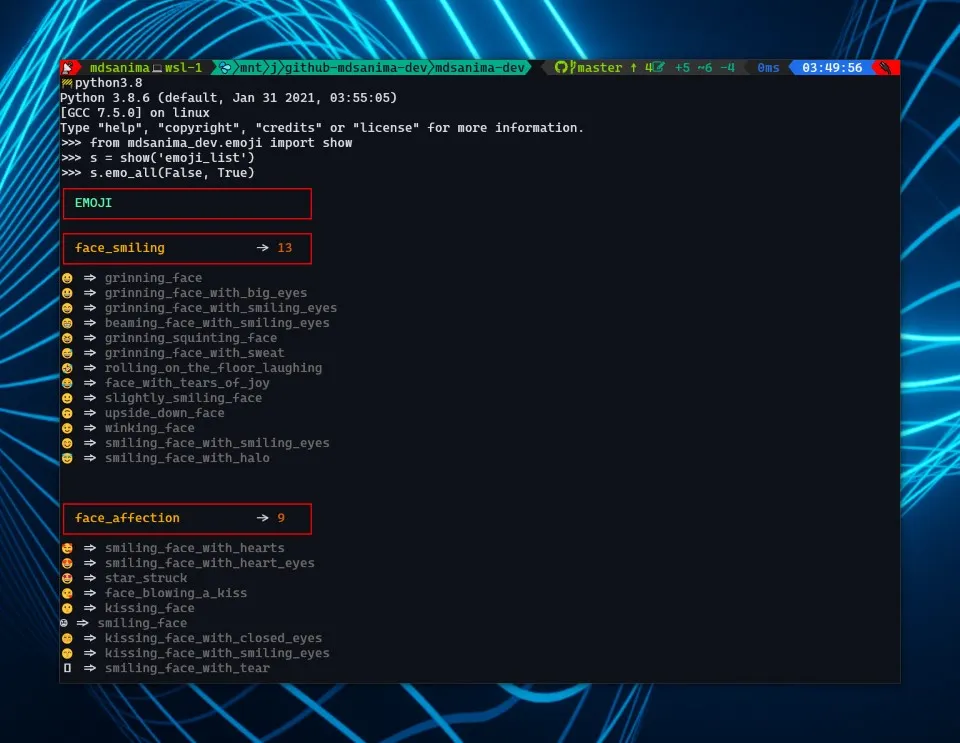
METHOD emo_all(True, True)
#
Both arguments are true so I will show us the number assigned to the emoji and its name. All emoticons in a given group will be displayed individually, each on a separate line:
from mdsanima_dev.emoji import show
s = show("emoji_list")
s.emo_all(True, True)
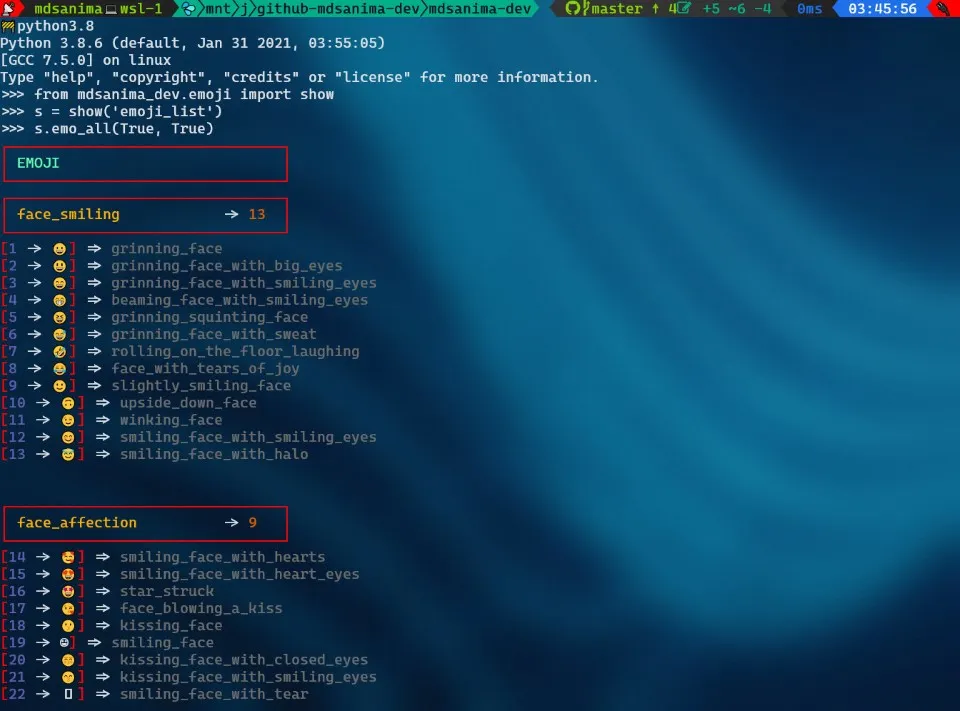
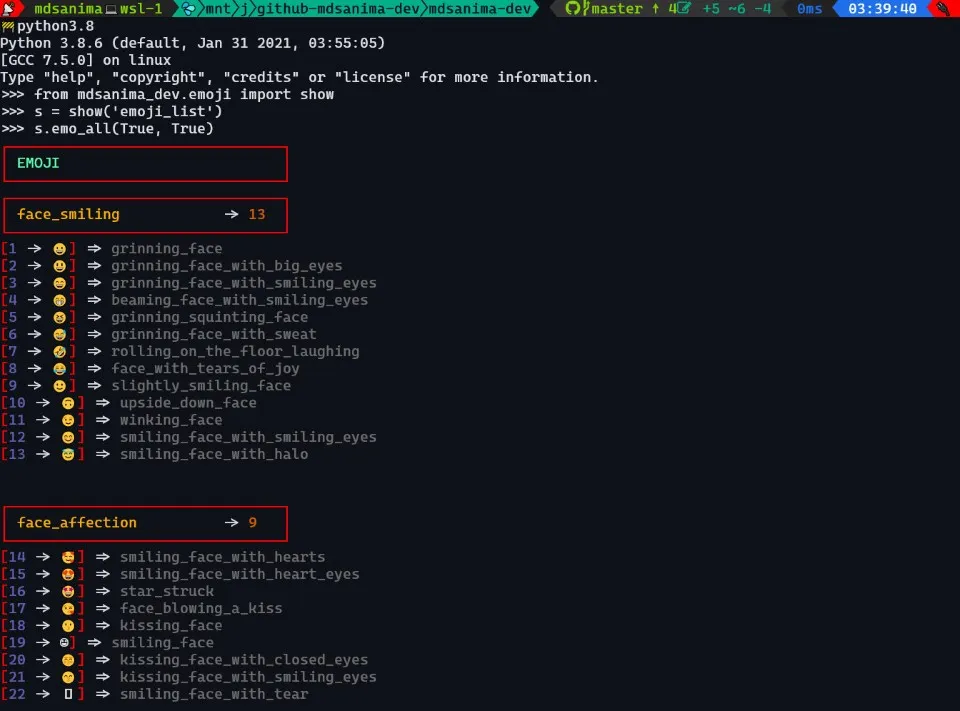
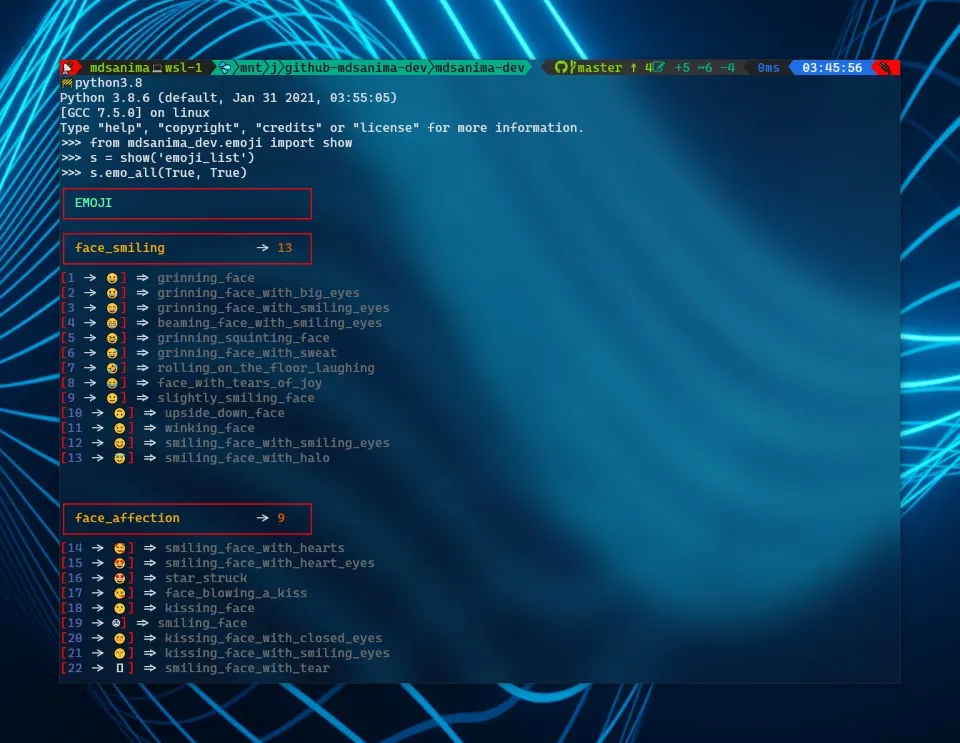
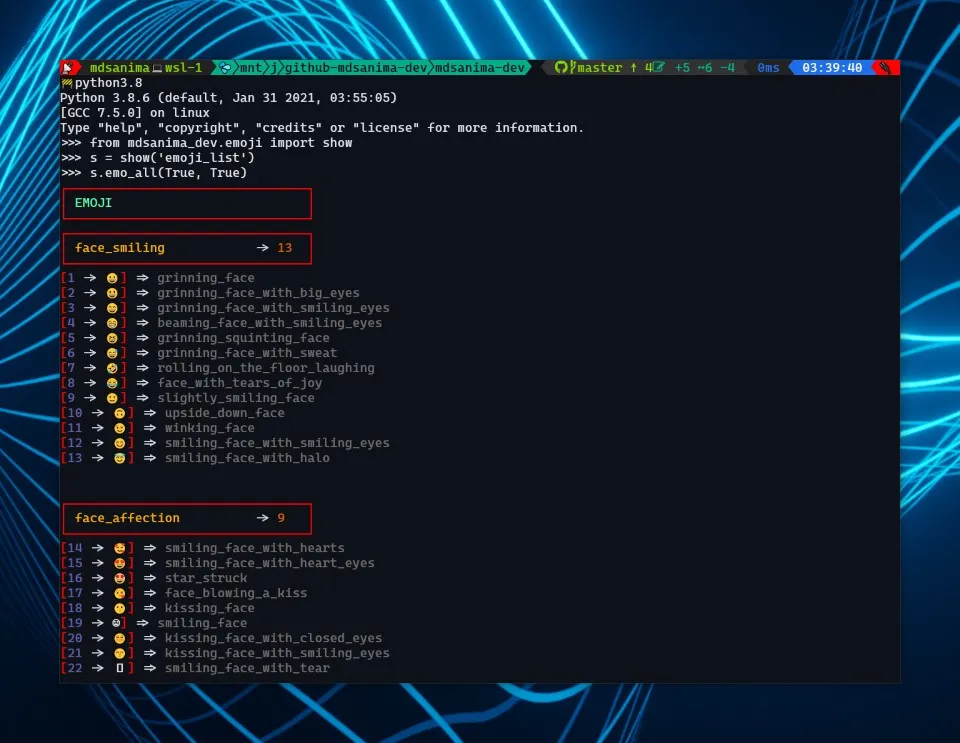
METHOD emo_big_head()
#
This method shows all available big heads from the list you’re selected:
from mdsanima_dev.emoji import show
s = show("emoji_list")
s.emo_big_head()
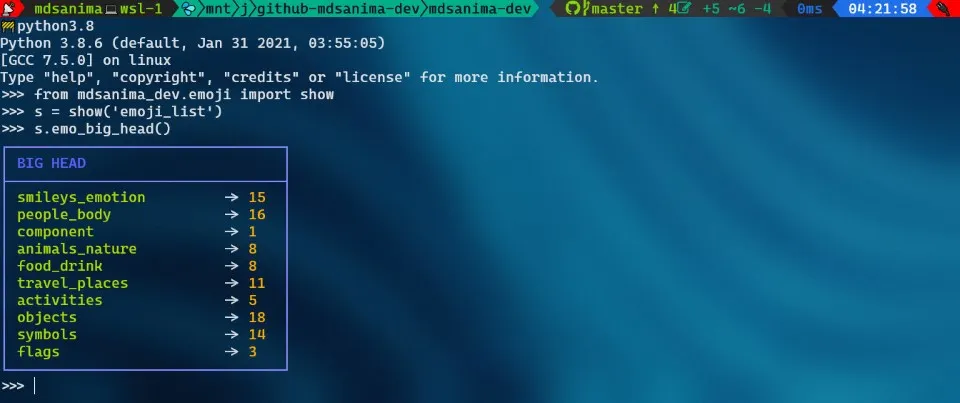
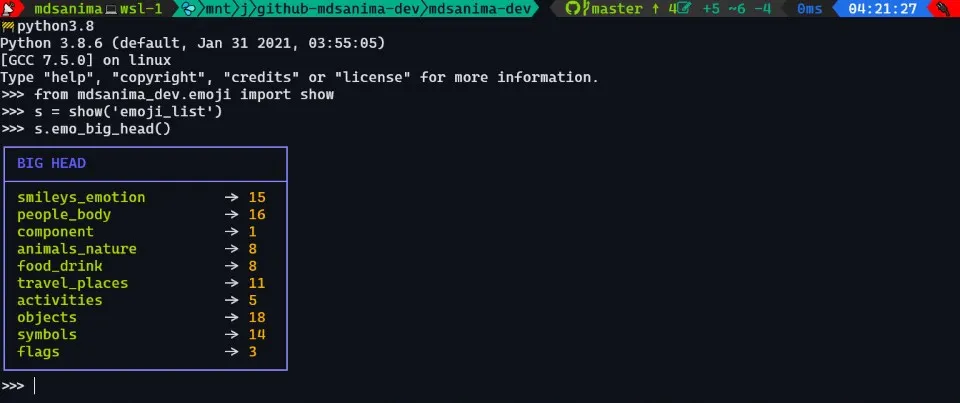
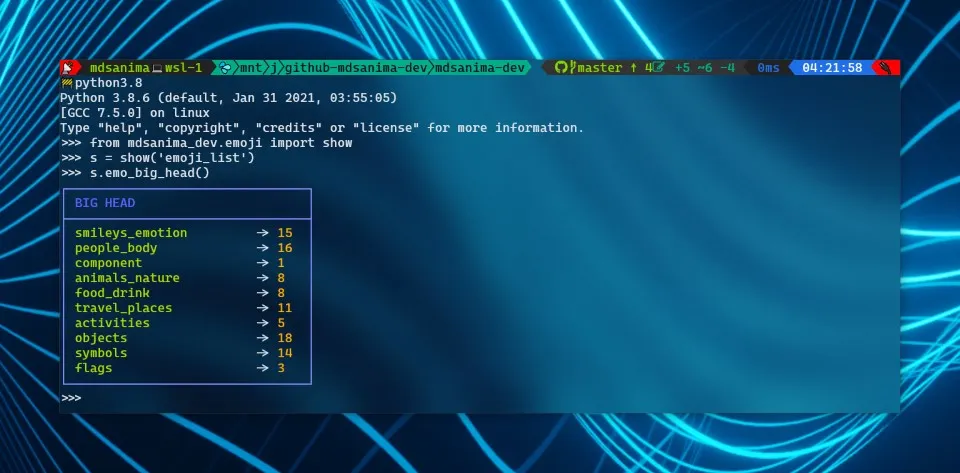
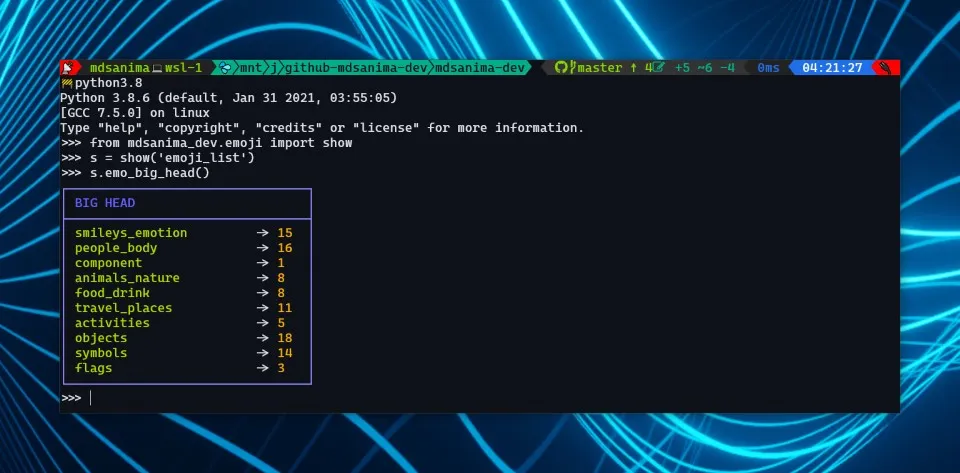
METHOD emo_head()
#
This method displays all emojis from a given big head and medium head. You always need to enter these two arguments big_head and medium_head. The next two arguments are for displaying emoji numbers and their names:
from mdsanima_dev.emoji import show
s = show("emoji_list")
s.emo_head("objects", "music")
s.emo_head("objects", "music", True, False)
s.emo_head("objects", "music", True, True)
s.emo_head("objects", "music", False, True)
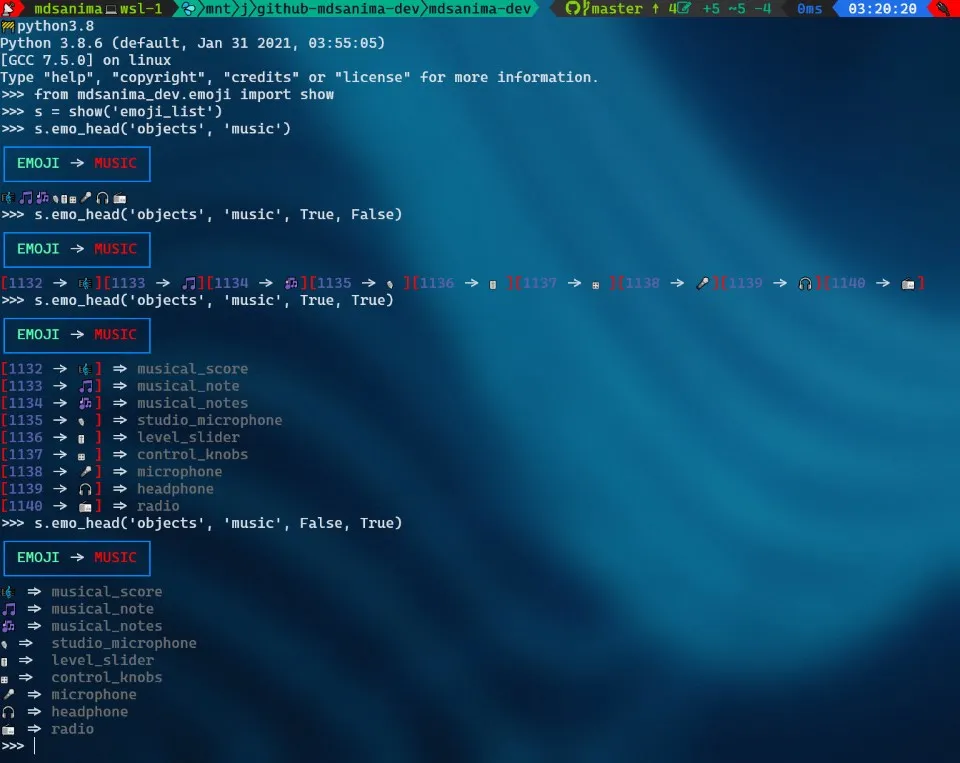
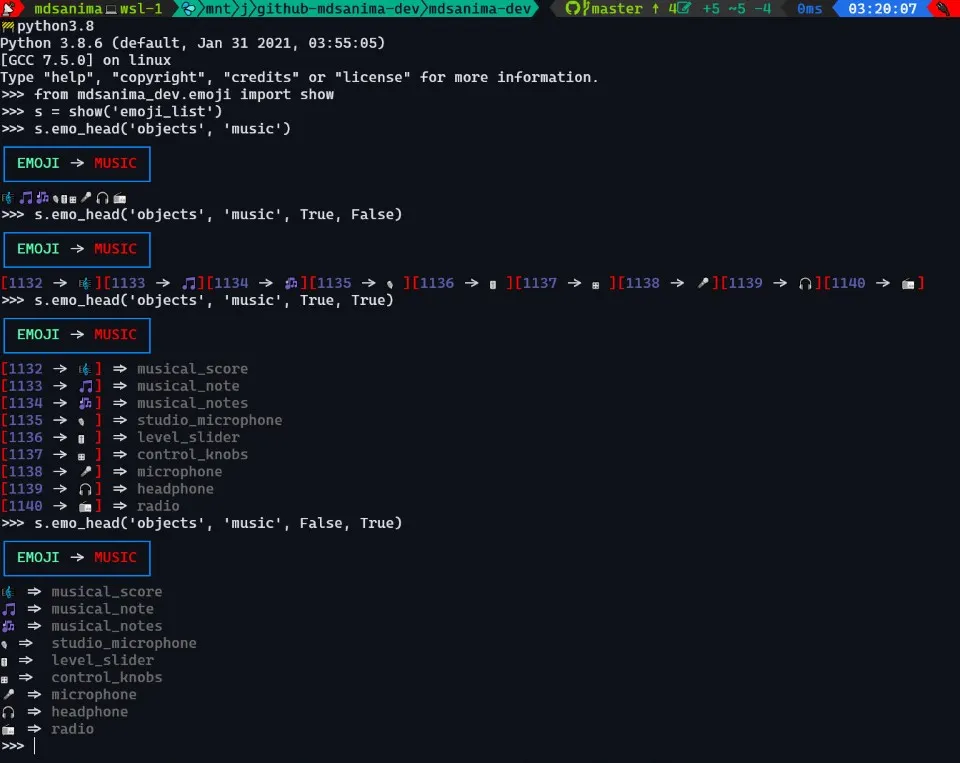
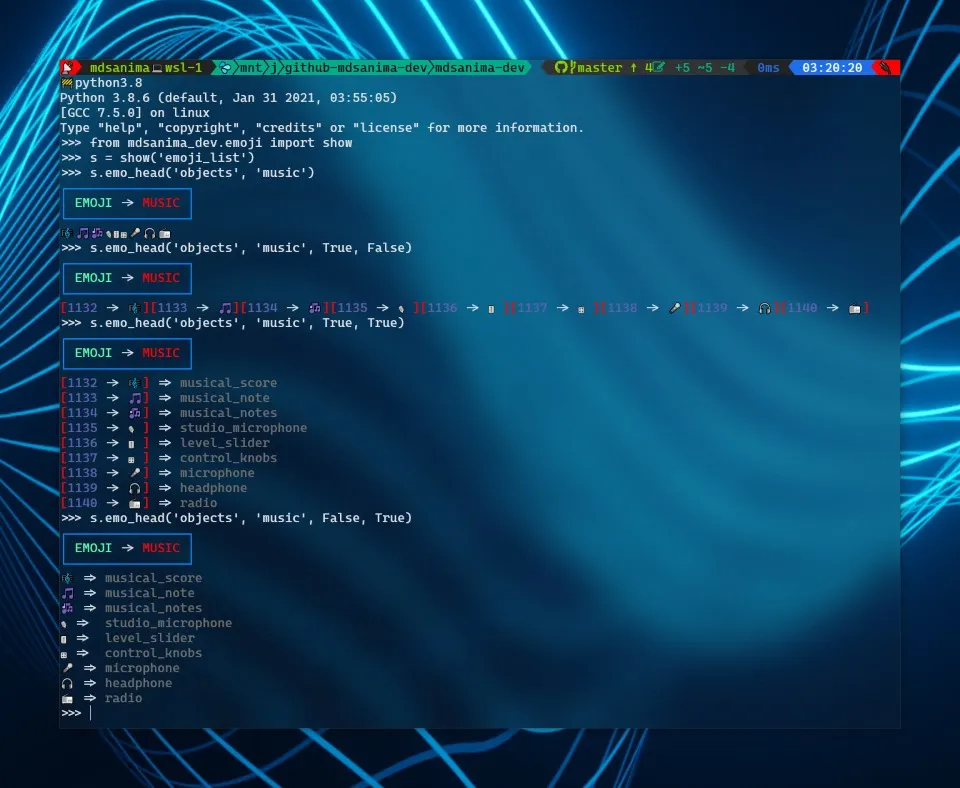
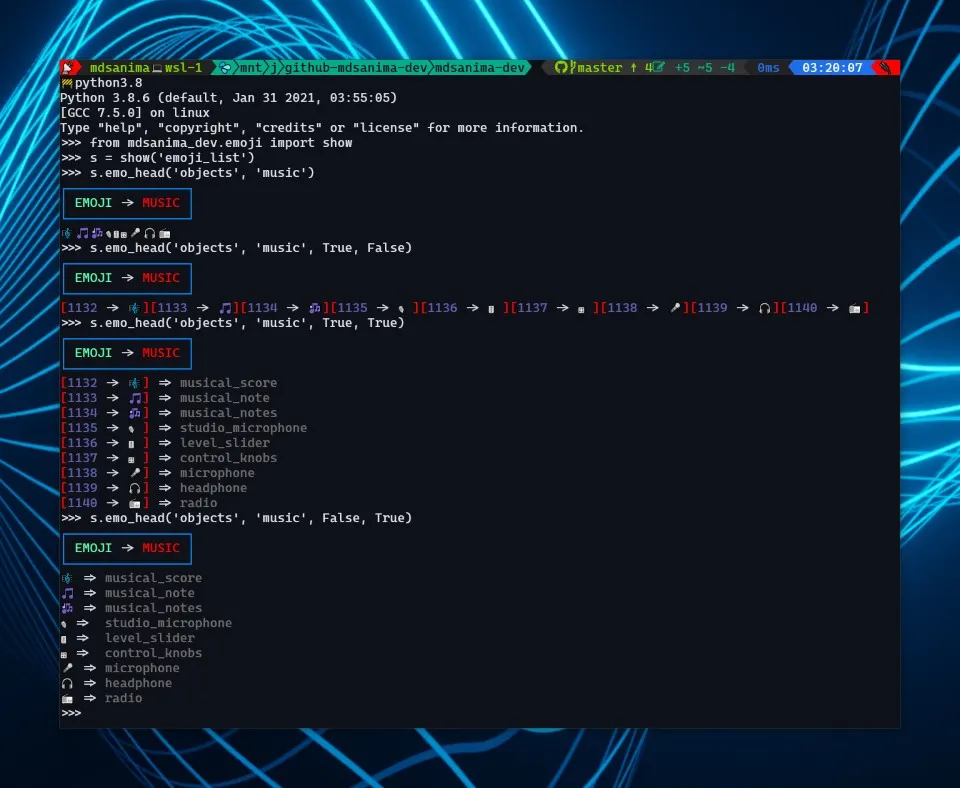
METHOD emo_medium_head()
#
This method shows all available medium heads from the list you’re selected:
from mdsanima_dev.emoji import show
s = show("emoji_list")
s.emo_medium_head()
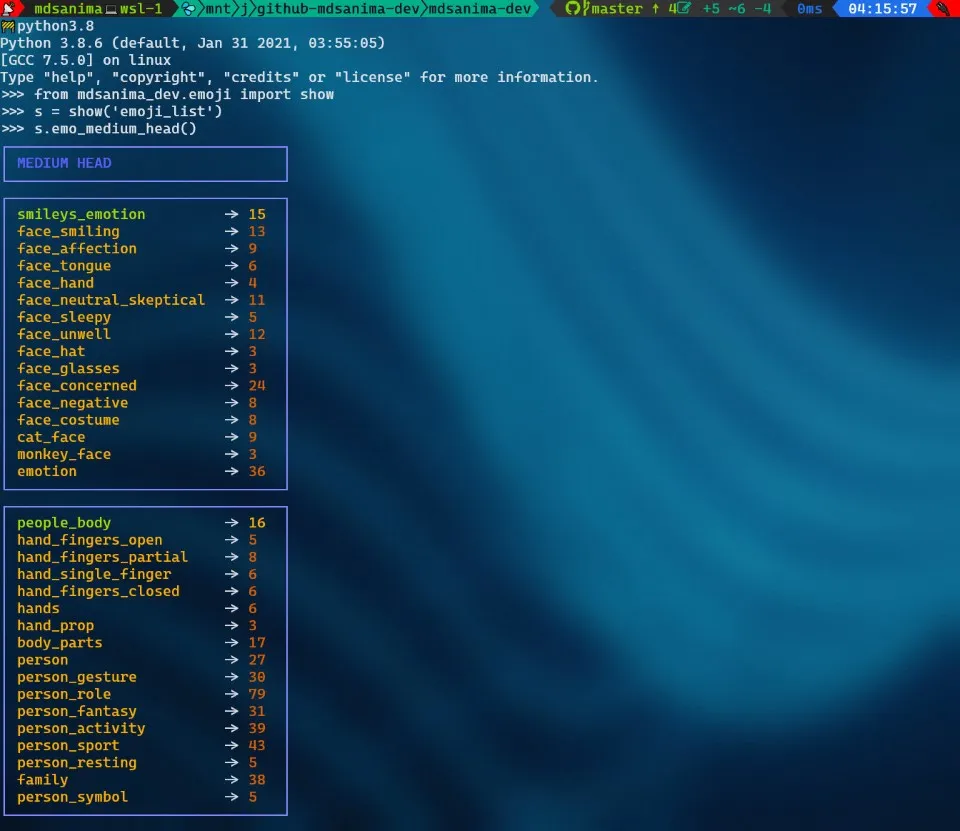
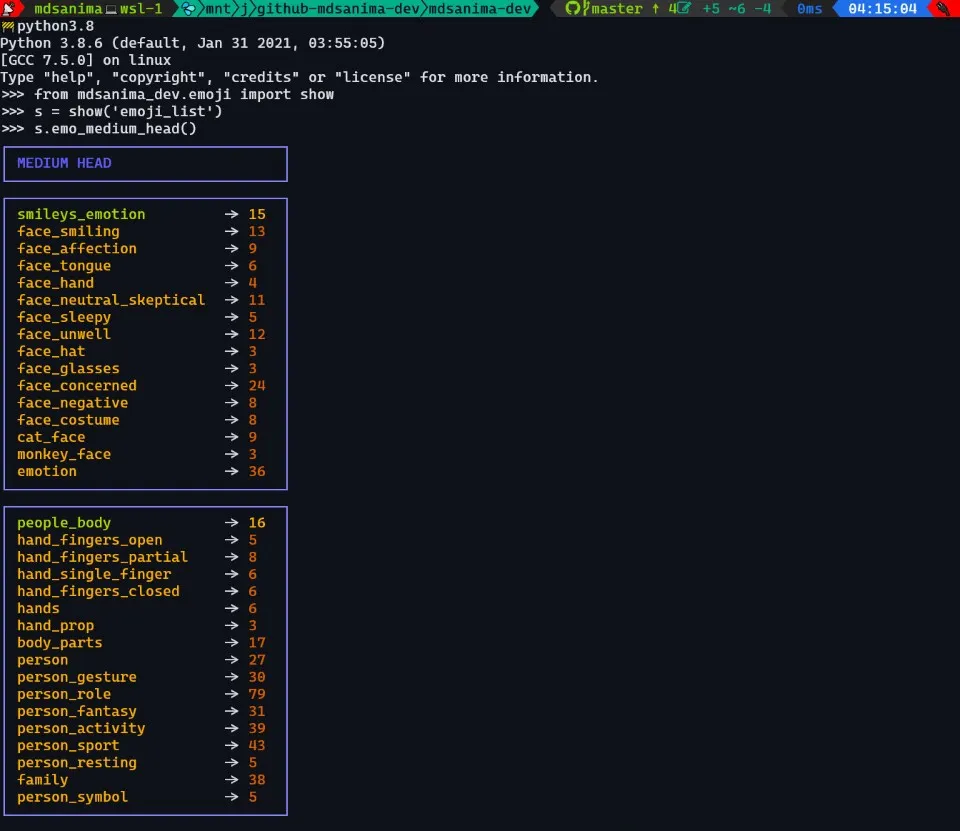
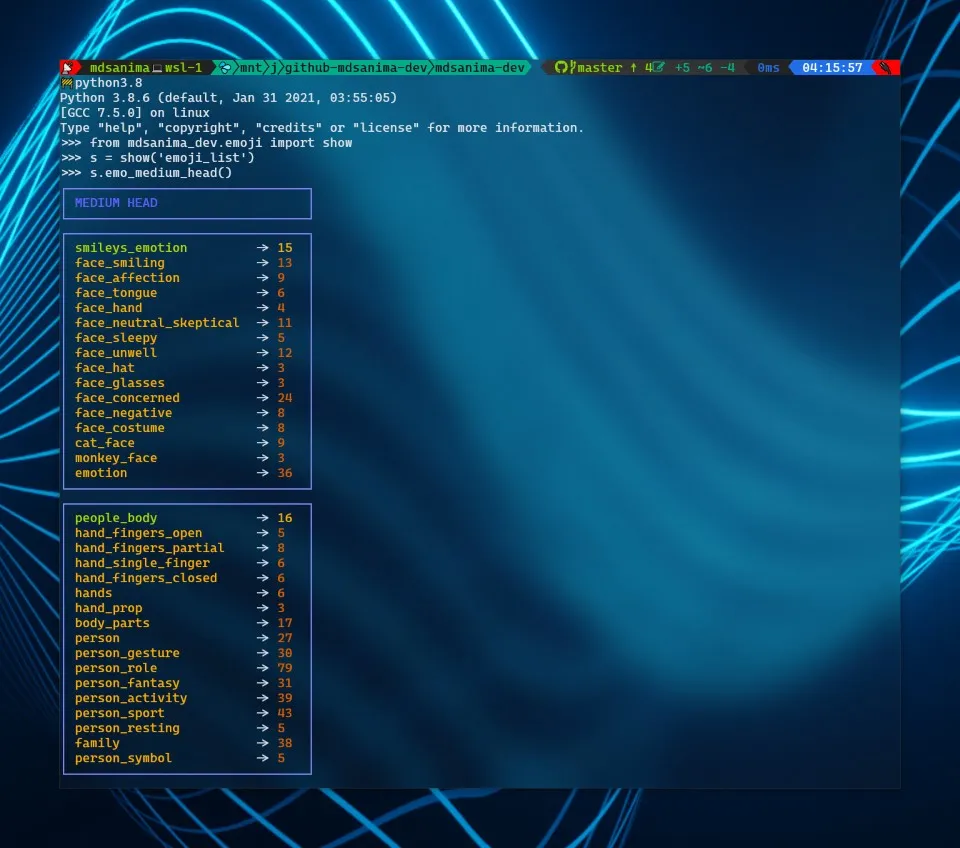
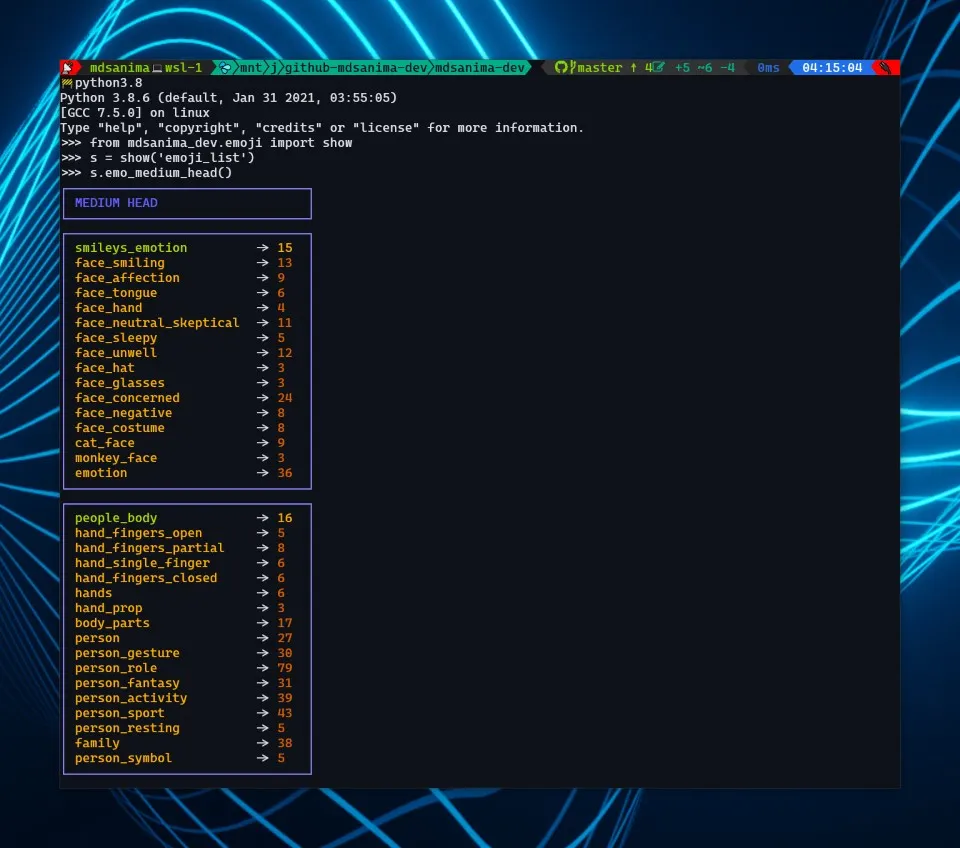
METHOD emoji()
#
Now you know what numbers each emoji has, so you can use this method for your
code. If you want to print on a new line use this argument end = "\n"
or just simple show("emoji_list").emoji(86, "\n")
thats it:
from mdsanima_dev.emoji import show
s = show("emoji_list")
s.emoji(1132)
s.emoji(14, "\n")
s.emoji(86, end="\n")
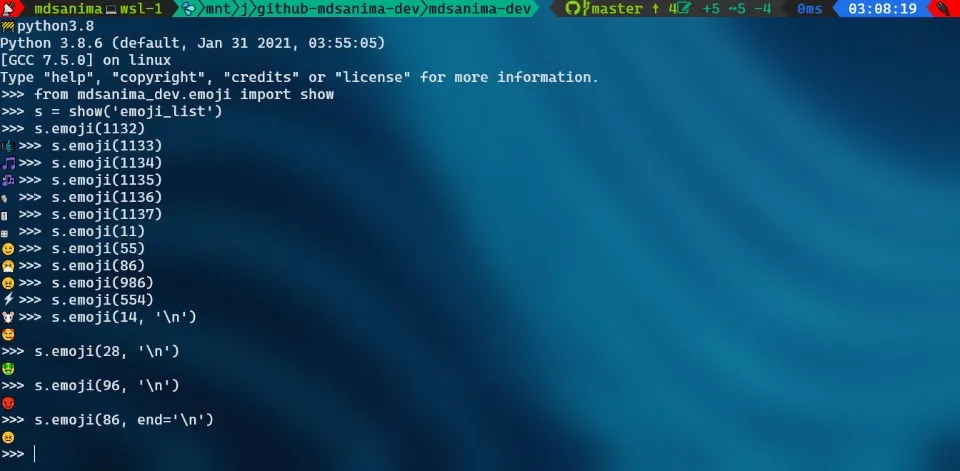
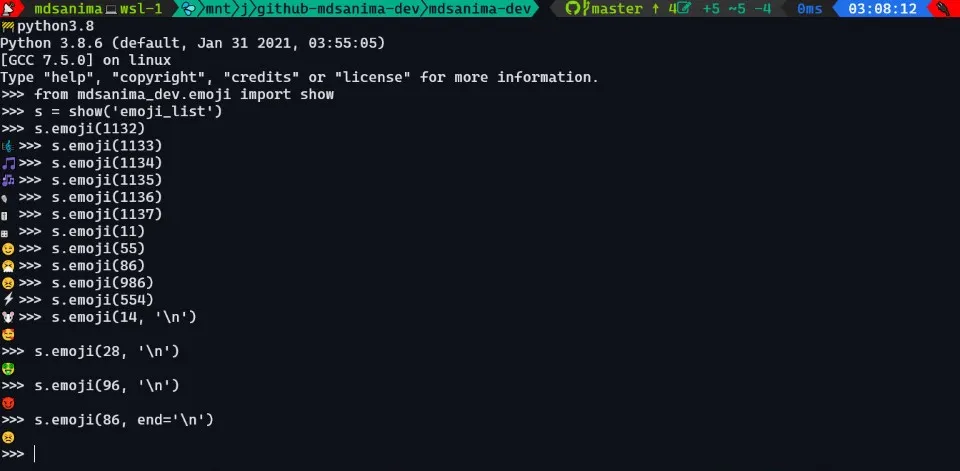
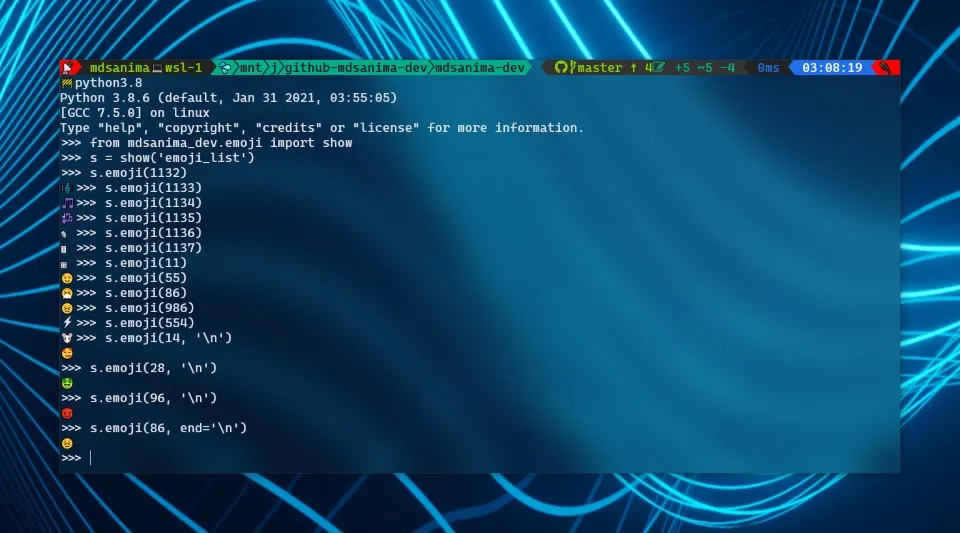
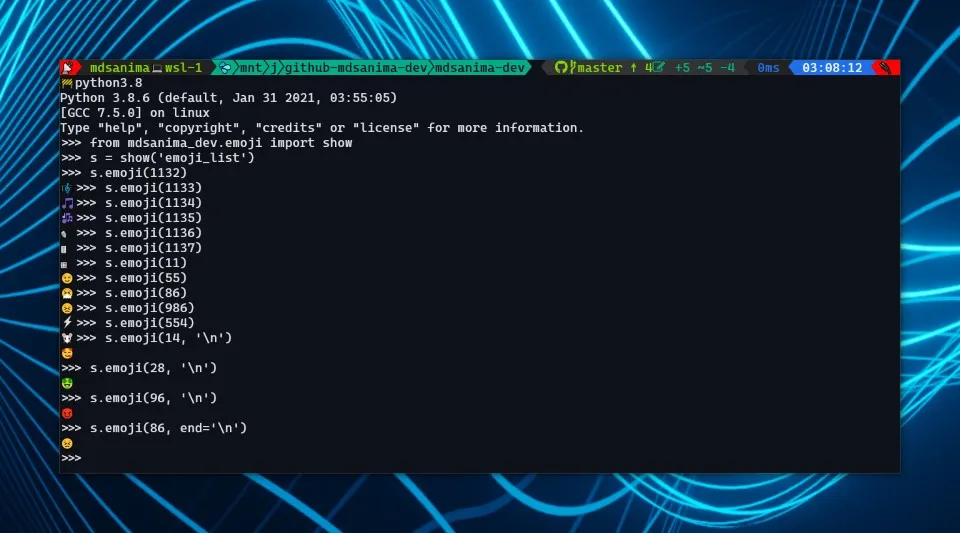
METHOD emoji()
FOR LOOP emoji_list
FIRST#
This way you can now print the first 67 emojis from the list selected:
from mdsanima_dev.emoji import show
s = show("emoji_list")
for i in range(1, 167):
s.emoji(i)
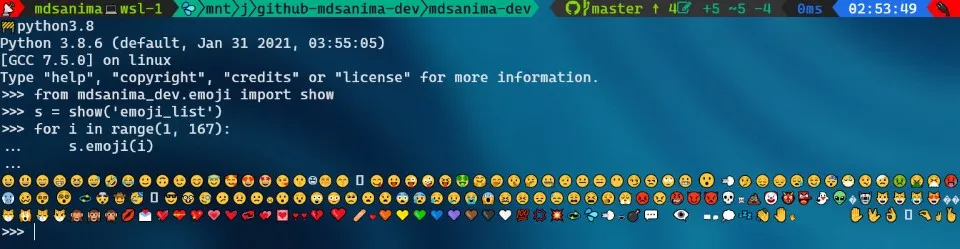
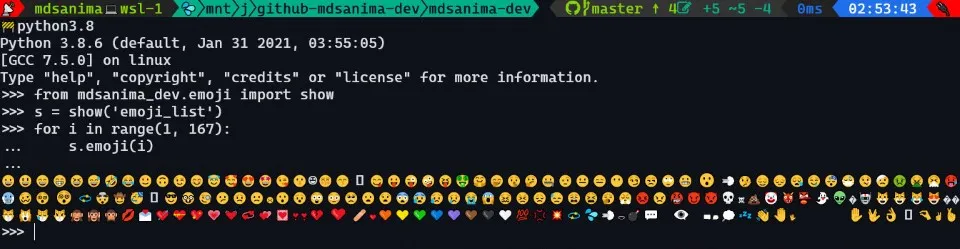
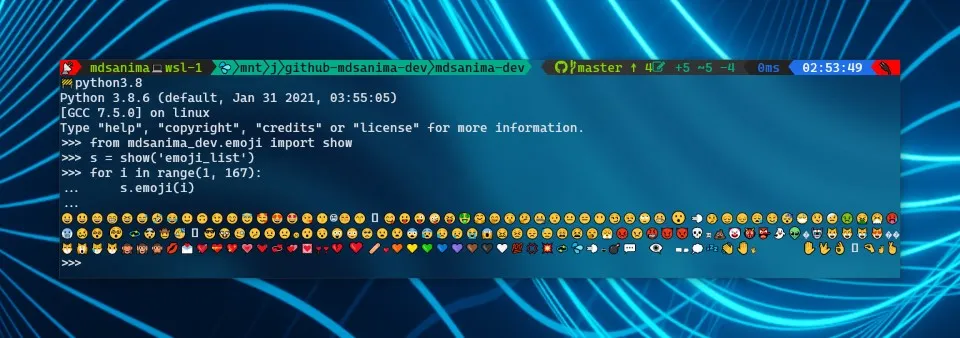
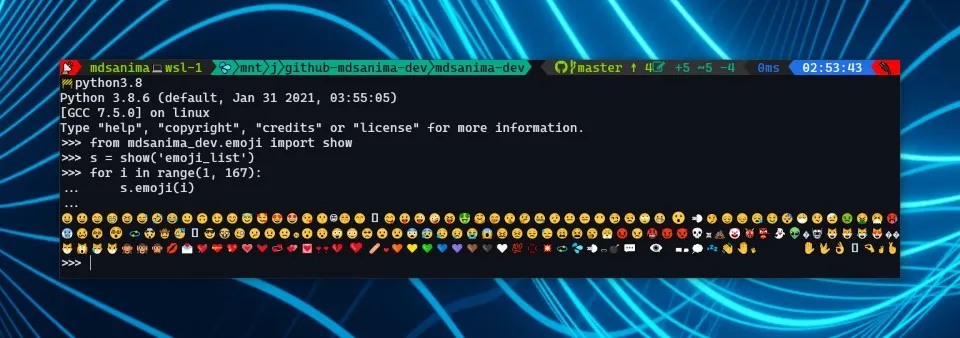
METHOD emoji()
FOR LOOP emoji_list
ALL#
This way you can print all available emojis from the list selected:
from mdsanima_dev.emoji import show
s = show("emoji_list")
for i in range(1, 1816):
s.emoji(i)
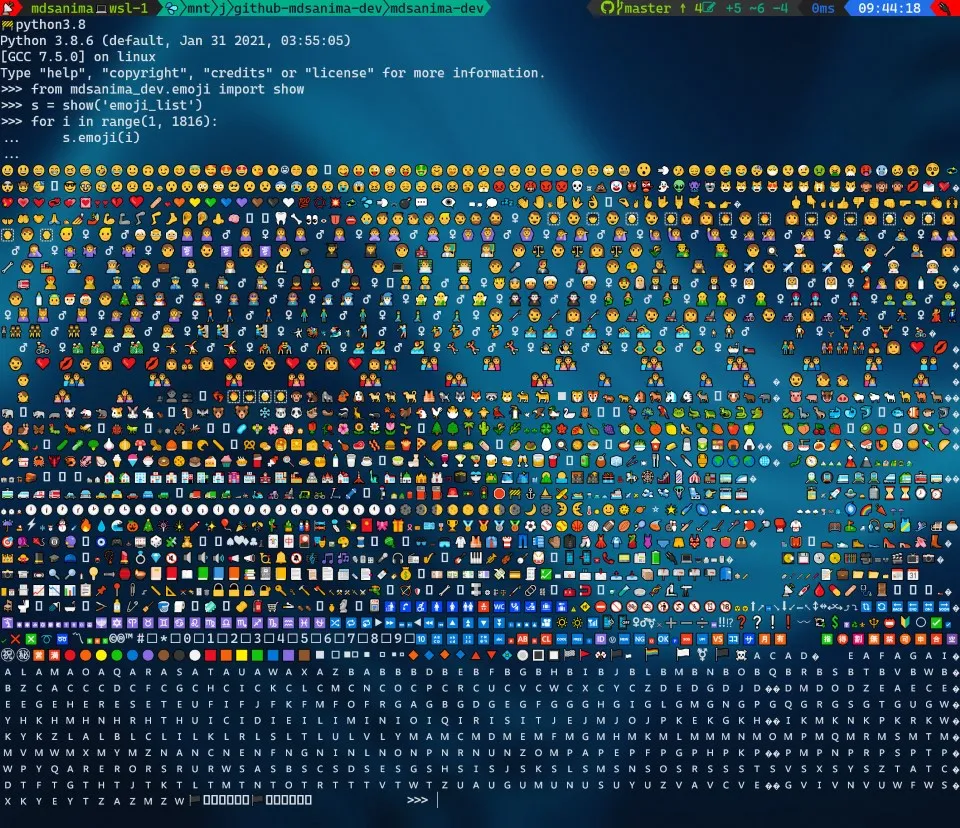
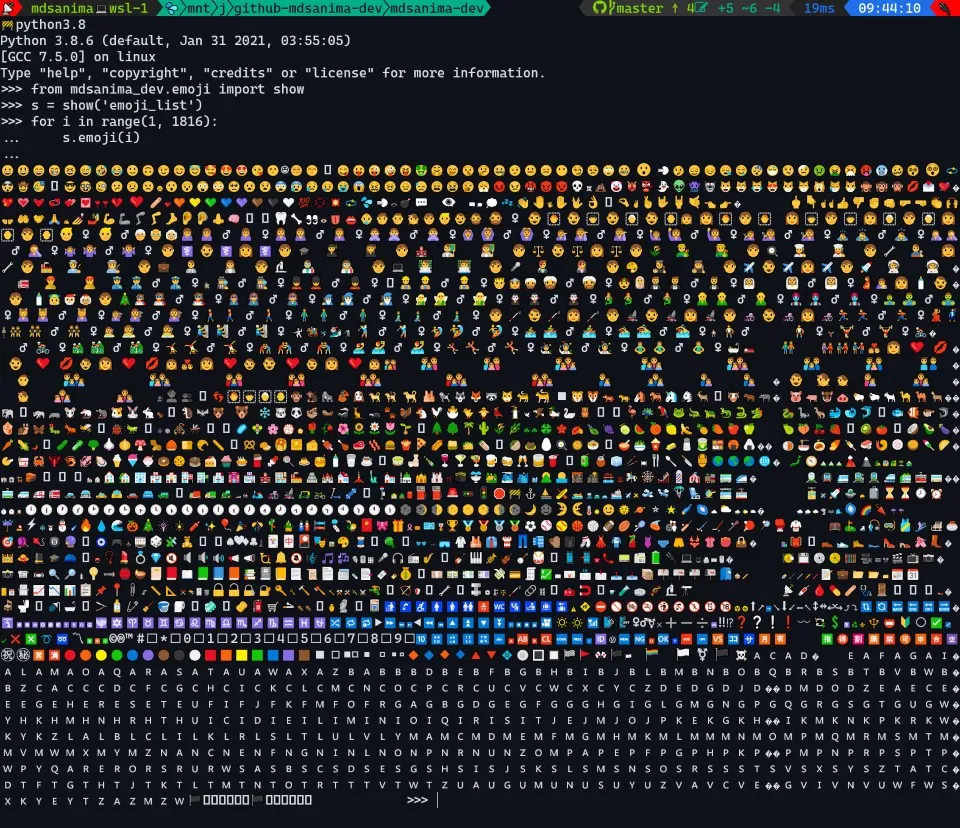
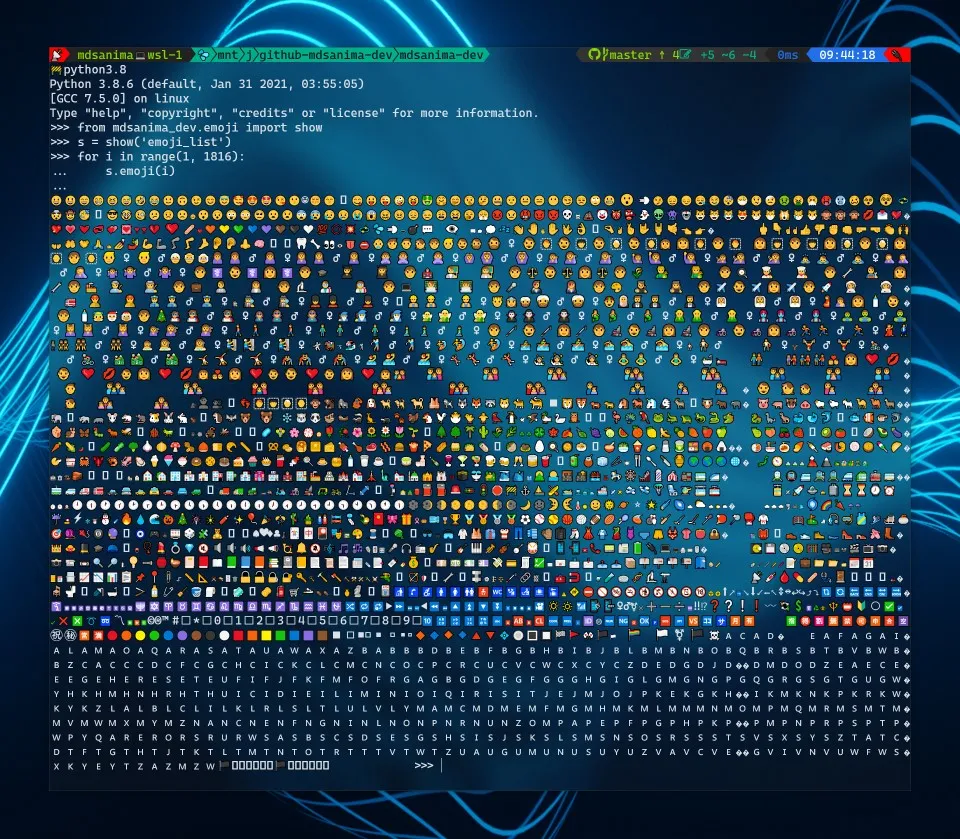
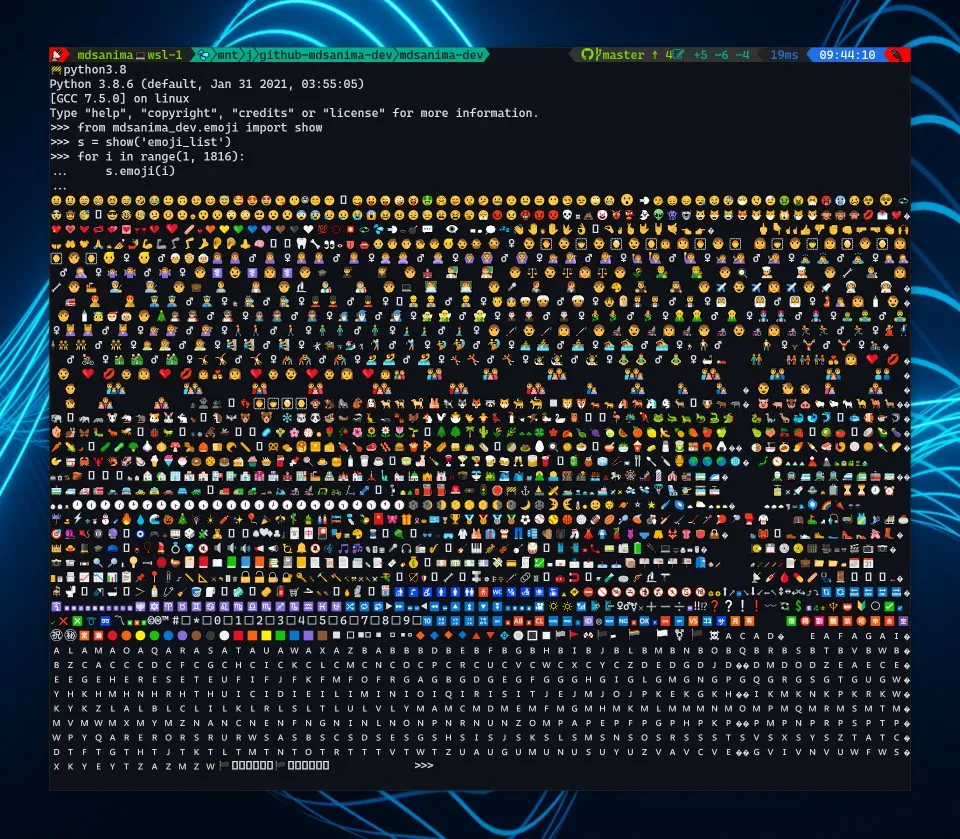
METHOD emoji()
FOR LOOP emoji_modifiers
ALL#
This way you can print all available emojis from the list selected:
from mdsanima_dev.emoji import show
s = show("emoji_modifiers")
for i in range(1, 1705):
s.emoji(i)
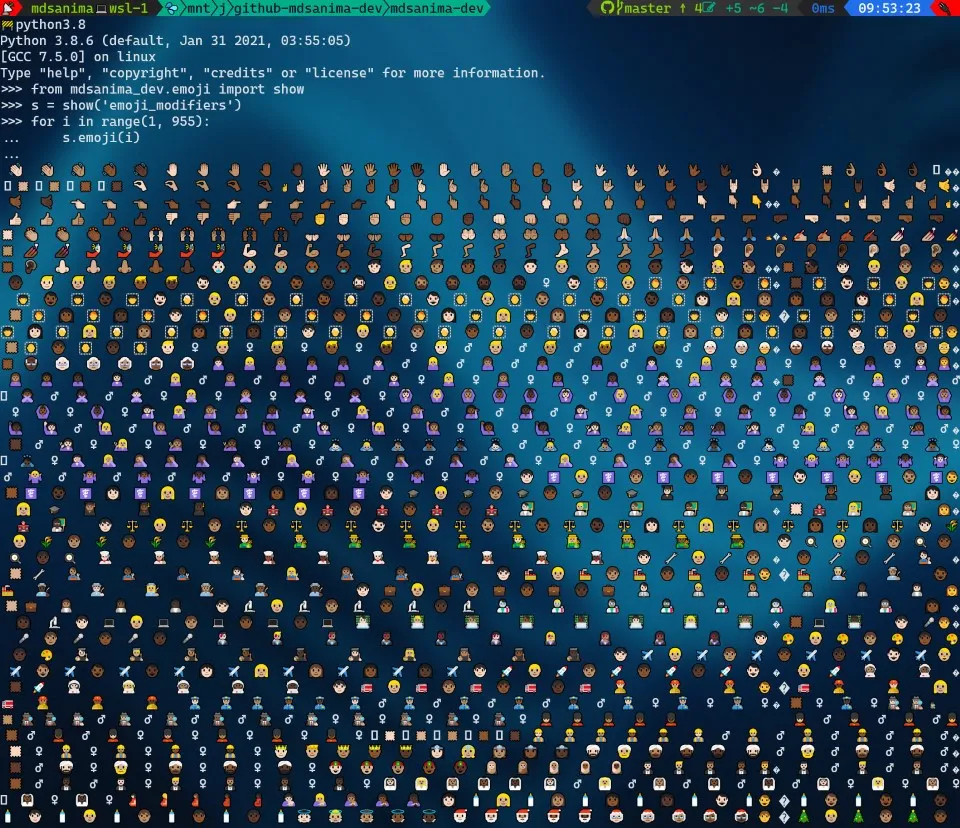
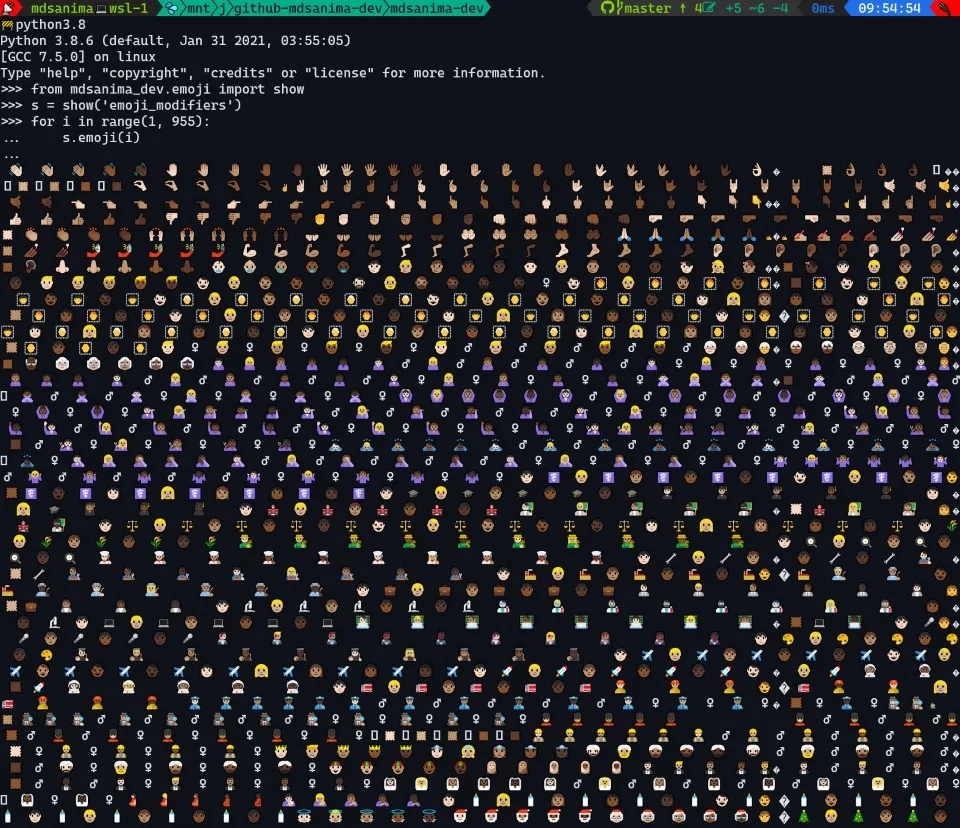
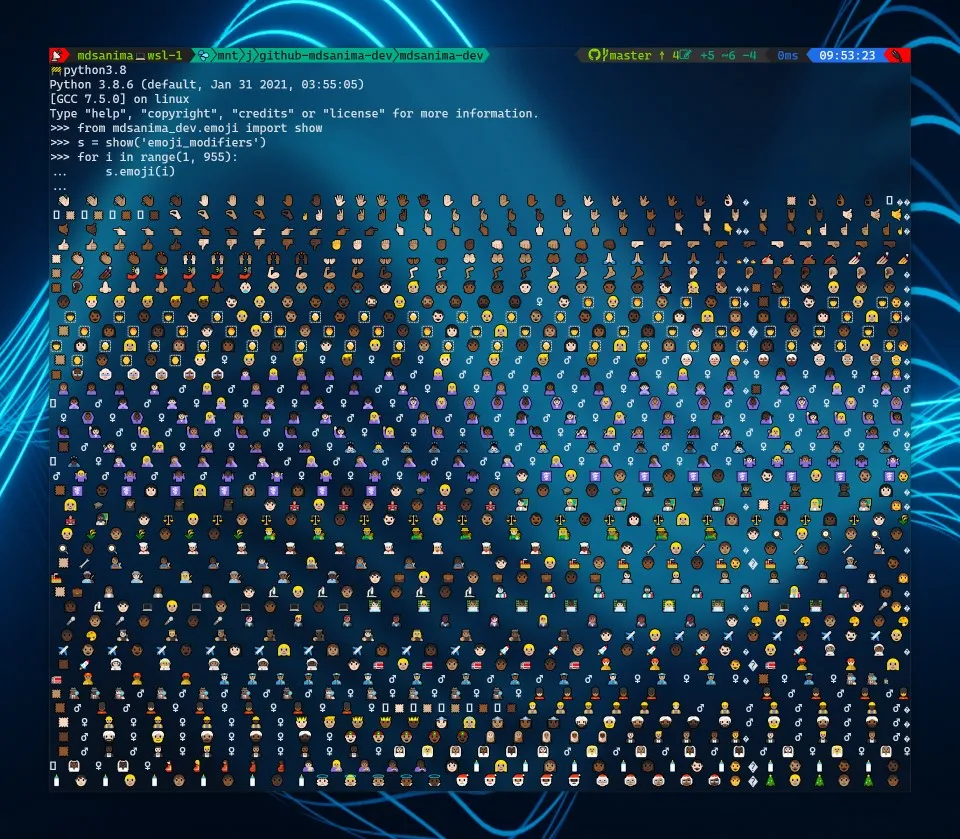
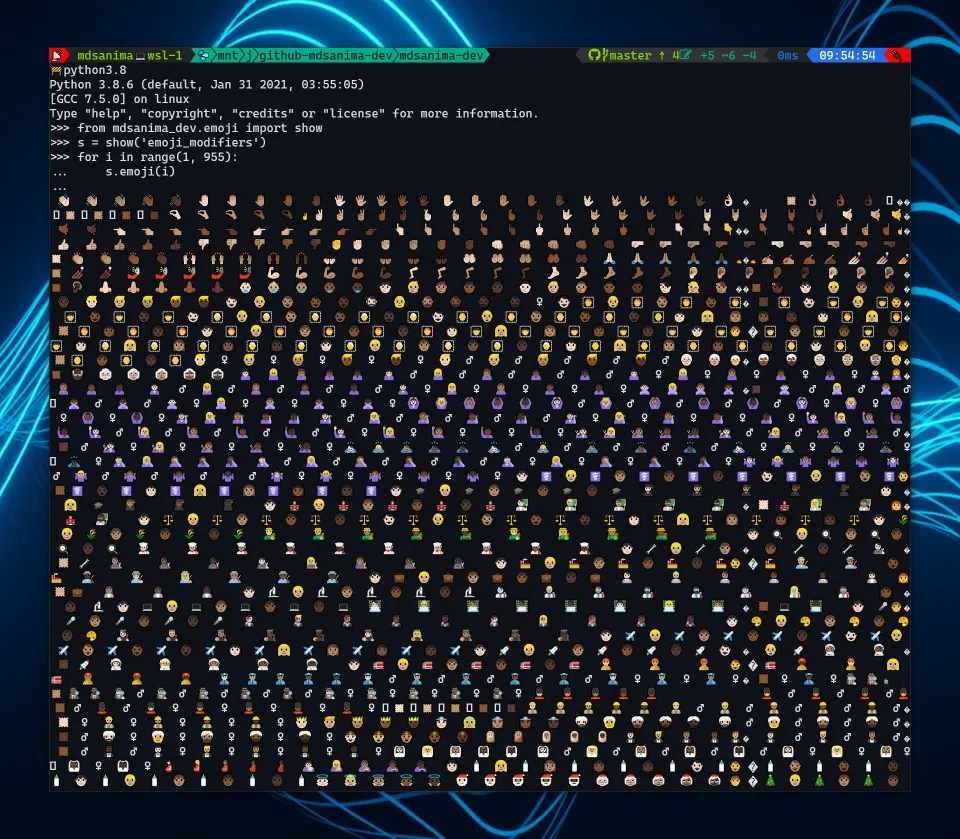
ALL METHOD
#
Show all methods to get the correct number for your emoji:
from mdsanima_dev.emoji import show
s = show("emoji_modifiers")
s.emo_big_head()
s.emo_medium_head()
s.emo_head("component", "skin_tone")
s.emo_head("component", "skin_tone", True, False)
s.emo_head("component", "skin_tone", False, True)
s.emo_head("component", "skin_tone", True, True)
s.emoji(1704, "\n")
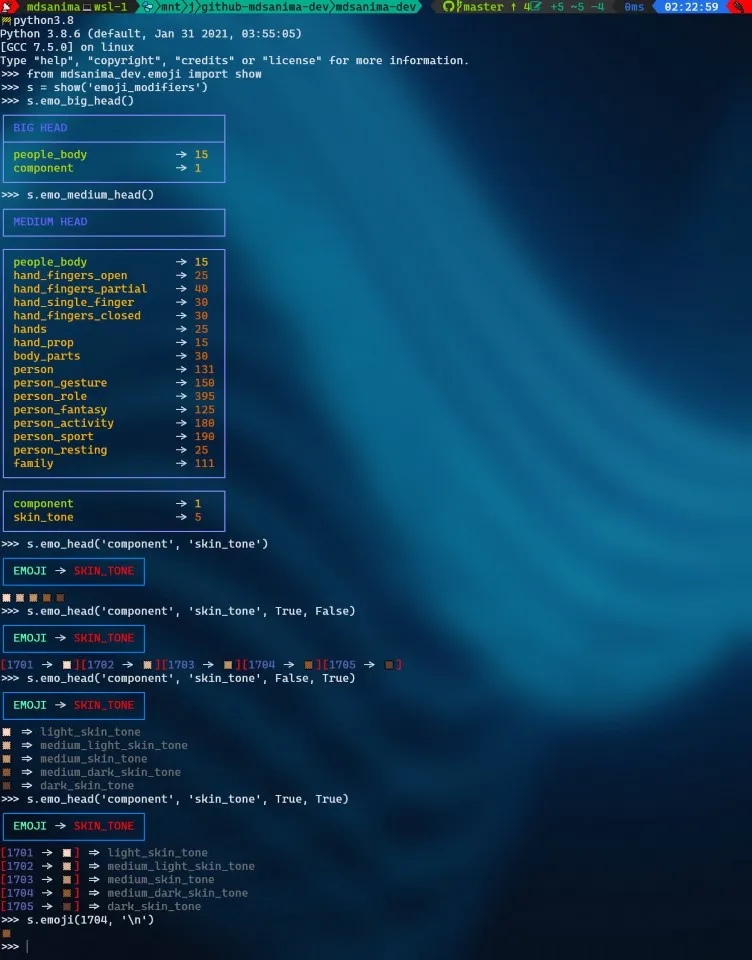
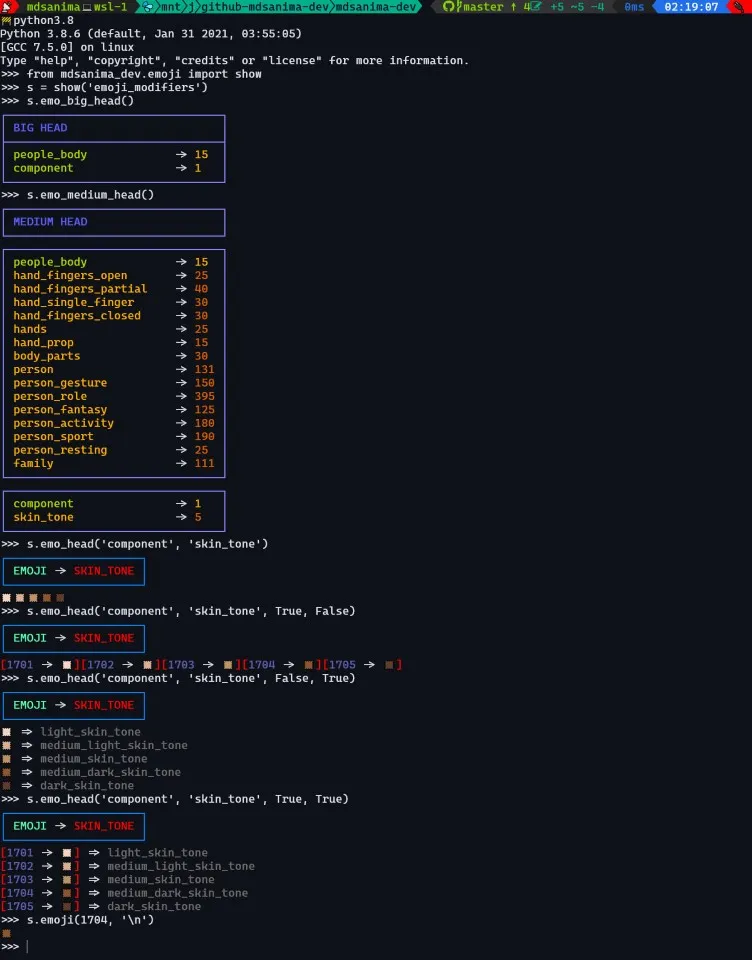
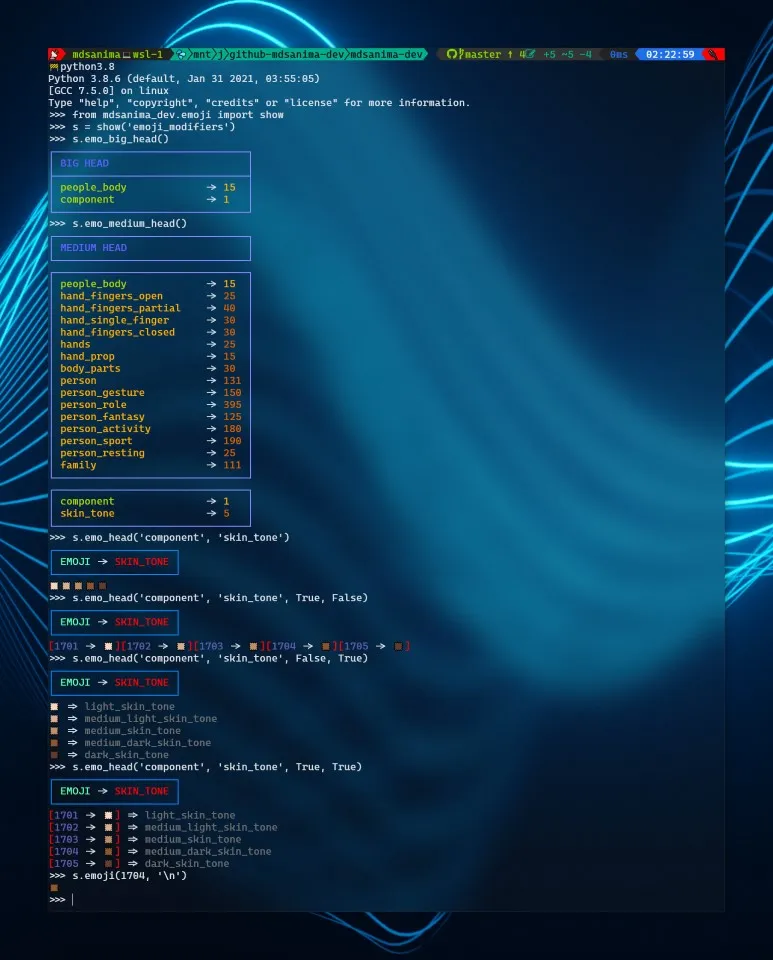
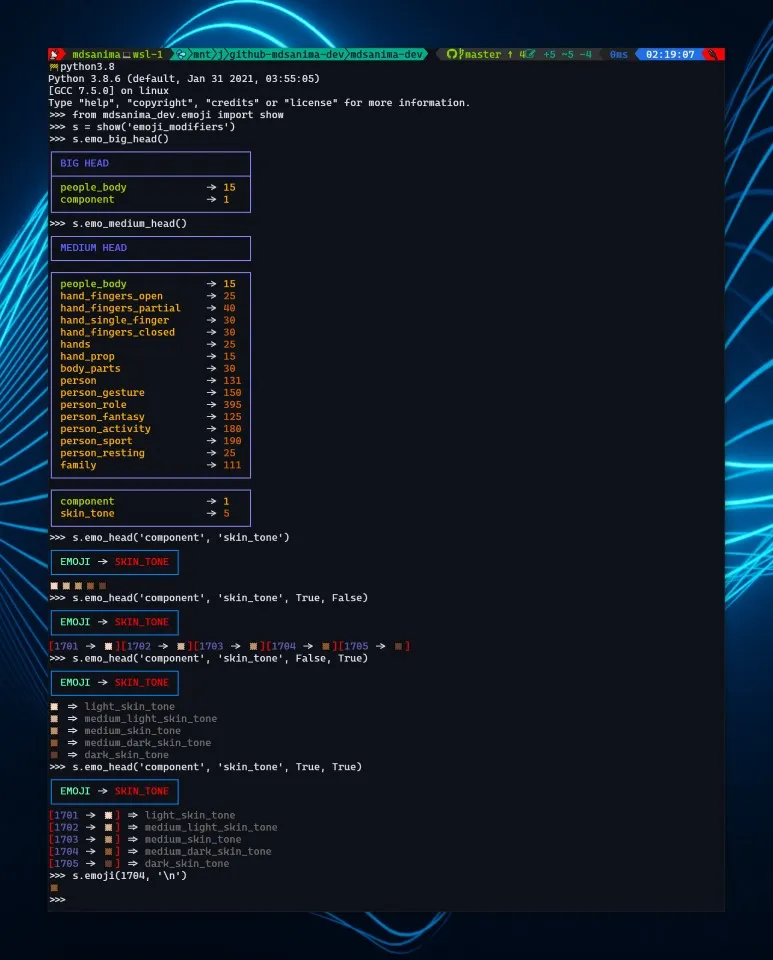